帮我写一个c++的贪心算法
时间: 2023-09-06 11:05:30 浏览: 106
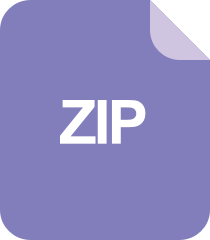
C++贪心算法.zip
### 回答1:
我可以给你一个简单的贪心算法的C语言代码:#include<stdio.h>
// Function to find the maximum for a array of size n
int findMax(int arr[], int n)
{
int max = arr[0];
for (int i = 1; i < n; i++)
if (arr[i] > max)
max = arr[i];
return max;
}
// Function to implement greedy algorithm
void greedy(int money[], int n)
{
// Sort the money array in descending order
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - 1 - i; j++) {
if (money[j] < money[j + 1]) {
int temp = money[j];
money[j] = money[j + 1];
money[j + 1] = temp;
}
}
}
int total = 0;
// Find the maximum money
int maxMoney = findMax(money, n);
while (maxMoney > 0) {
// Greedily subtract maximum money from
// each element
for (int i = 0; i < n; i++) {
if (money[i] != 0) {
money[i] -= maxMoney;
total += maxMoney;
}
}
// Find the new maximum money
maxMoney = findMax(money, n);
}
printf("Total money collected by Greedy algorithm = %d", total);
}
// Driver program to test above function
int main()
{
int money[] = {10, 5, 7, 8};
int n = sizeof(money) / sizeof(money[0]);
greedy(money, n);
return 0;
}
### 回答2:
C语言的贪心算法通常用来解决优化问题,其核心思想是每一步都选择当前情况下的局部最优解,希望通过一系列贪心选择达到全局最优解。
下面我以找零钱问题为例,给出一个贪心算法的C语言实现:
```c
#include <stdio.h>
void greedyChange(int coins[], int n, int amount) {
int count = 0;
// 从面额最大的硬币开始选择
for (int i = n - 1; i >= 0; i--) {
// 尽量选择多个当前面额的硬币
while (amount >= coins[i]) {
amount -= coins[i];
count++;
}
}
if (amount == 0) {
printf("需要的硬币数量为:%d\n", count);
} else {
printf("无法找零\n");
}
}
int main() {
int coins[] = {10, 5, 1};
int n = sizeof(coins) / sizeof(coins[0]);
int amount = 25;
greedyChange(coins, n, amount);
return 0;
}
```
上述代码中,我们假设有10、5和1三种面额的硬币,需要找零25元。算法从面额最大的硬币10元开始选择,尽量选择多个10元硬币,当无法再选择10元硬币后,转而选择面额为5元的硬币,同样尽量选择多个。最后选择面额为1元的硬币。整个过程中,每一步都是局部最优解,最终达到全局最优解。
阅读全文
相关推荐
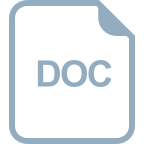
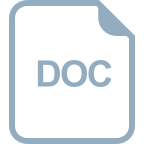
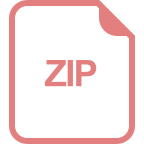
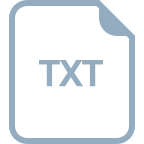
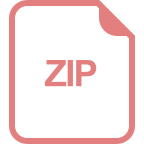
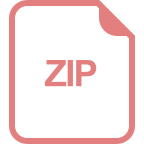
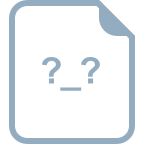
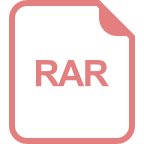
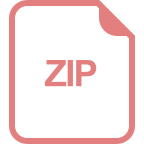
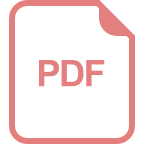
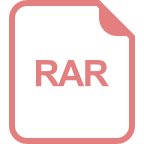
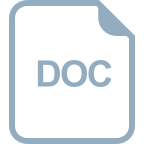
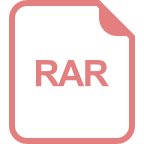
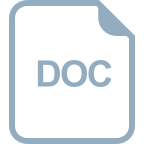