python如何打印字典
时间: 2023-07-05 16:30:01 浏览: 55
可以使用 `print()` 函数来打印字典,示例如下:
```python
my_dict = {'apple': 'fruit', 'carrot': 'vegetable', 'orange': 'fruit', 'broccoli': 'vegetable'}
# 打印整个字典
print(my_dict)
# 打印字典中某个键对应的值
print(my_dict['apple'])
```
上面的代码将整个字典打印出来,以及打印了字典中键 `apple` 对应的值。运行上面的代码会得到如下输出:
```
{'apple': 'fruit', 'carrot': 'vegetable', 'orange': 'fruit', 'broccoli': 'vegetable'}
fruit
```
注意,字典是无序的,因此打印出来的顺序可能和定义时的顺序不同。如果需要按照一定的顺序打印字典,可以先将字典转换为列表,然后按照需要的顺序打印。
相关问题
python打印字典
以下是Python打印字典的几种方法:
1. 使用print()函数打印整个字典
```python
dict = {'name': 'John', 'age': 25, 'city': 'New York'}
print(dict) # 输出:{'name': 'John', 'age': 25, 'city': 'New York'}
```
2. 使用for循环遍历字典的键和值,并打印出来
```python
dict = {'name': 'John', 'age': 25, 'city': 'New York'}
for key, value in dict.items():
print(key + ": " + str(value))
# 输出:
# name: John
# age: 25
# city: New York
```
3. 使用for循环遍历字典的键,并打印出键和对应的值
```python
dict = {'name': 'John', 'age': 25, 'city': 'New York'}
for key in dict.keys():
print(key)
print(dict[key])
# 输出:
# name
# John
# age
# 25
# city
# New York
```
python 打印字典
可以使用 Python 的 `print()` 函数来打印字典。以下是一个简单的示例:
```python
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
print(my_dict)
```
输出:
```
{'name': 'Alice', 'age': 25, 'city': 'New York'}
```
你也可以使用循环遍历字典中的键和值,然后将它们打印出来:
```python
for key, value in my_dict.items():
print(key, ":", value)
```
输出:
```
name : Alice
age : 25
city : New York
```
相关推荐
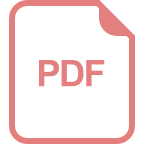
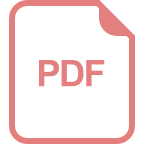
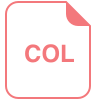
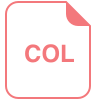
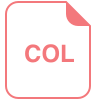
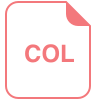
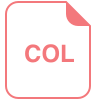







