用java编写一个程序,识别英文句子中的单词。"The shortest answer is doing." 分析: 字符串对象的split()方法可以按照指定内容将字符串分割为数组
时间: 2024-12-19 12:24:04 浏览: 1
在Java中,你可以使用`String`类的`split()`方法来识别英文句子中的单词。这个方法会根据给定的分隔符(默认为空格、逗号、分号等)将字符串拆分成一个单词数组。下面是一个简单的例子:
```java
public class WordIdentifier {
public static void main(String[] args) {
String sentence = "The shortest answer is doing.";
// 使用空格作为分隔符,split()会返回一个包含每个单词的字符串数组
String[] words = sentence.split(" ");
for (int i = 0; i < words.length; i++) {
System.out.println("Word " + (i + 1) + ": " + words[i]);
}
}
}
```
运行这个程序,你会得到以下输出:
```
Word 1: The
Word 2: shortest
Word 3: answer
Word 4: is
Word 5: doing.
```
相关问题
输入一段英文(标点符号只有叹号,问号,句号,逗号),找出所有单词,去除重复单词后(不区分大小写,如And和and是同一单词),按字典序排序输出所有单词。 提示:1)使用字符串的lower()方法将输入字符串全部转换为小写; 2)使用字符串的replace()方法把标点符号替换成空格,再使用split()切分单词 输入:一段英文 输出:每行一个单词 输入样例: The shortest way to do many things is to only one thing at a time. 输出样例: a at do is many one only shortest the thing things time to way
好的,针对你的问题,我可以给出以下 Python 代码实现:
```python
text = input().lower() # 读入一行英文并转换为小写
for punc in '.,?!': # 去除标点符号
text = text.replace(punc, ' ')
words = set(text.split()) # 切分单词并去重
for word in sorted(words): # 排序并输出单词
print(word)
```
这段代码先读入一行英文,并使用字符串的 `lower()` 方法将其全部转换为小写。然后使用字符串的 `replace()` 方法将标点符号替换为空格,并使用 `split()` 方法切分出单词列表。由于要去重并按字典序排序输出,可以将单词列表转换为集合,再使用 `sorted()` 方法排序,最后逐个输出。
Consider a problem to find the shortest path from a given starting node s to any other nodes in the (undirected) graph. The length of a path in the graph is the number of edges included. Complete the function shortest_path(adj_list, s), which takes the adjacency list of the graph and a starting node as input, and returns a list containing the length of the shortest path from the starting node to all other nodes. Note: If there is no path from the starting node to a node, set the length of shortest path as float('inf'). You can use any Python built-in package to implement the stack/queue/priority queue if needed. Hint: Consider the three graph traversal strategies (DFS, BFS and PFS) discussed in the class. Which one is applicable in this problem? For example: Test Result adj_list = [[], [2, 3], [1, 4], [1], [2]] d = shortest_path(adj_list, 0) print(d) d = shortest_path(adj_list, 2) print(d) [0, inf, inf, inf, inf] [inf, 1, 0, 2, 1]
To find the shortest path from a given starting node s to any other nodes in the (undirected) graph, we can use Breadth-First Search (BFS) algorithm. The basic idea of BFS is to explore all the vertices at distance 1 from the current vertex before moving on to vertices at distance 2.
Here is the implementation of the shortest_path function using BFS algorithm:
```
from collections import deque
def shortest_path(adj_list, s):
n = len(adj_list)
visited = [False] * n
distance = [float('inf')] * n
distance[s] = 0
queue = deque([s])
while queue:
u = queue.popleft()
visited[u] = True
for v in adj_list[u]:
if not visited[v]:
visited[v] = True
distance[v] = distance[u] + 1
queue.append(v)
for i in range(n):
if not visited[i]:
distance[i] = float('inf')
return distance
```
In the above code, we first initialize two lists: visited and distance. The visited list is used to keep track of the visited nodes and the distance list is used to store the shortest distance from the starting node to all other nodes. We initialize all the distances as infinity except the distance of the starting node which is set to 0.
We then use a deque (double-ended queue) to implement the BFS algorithm. We start by adding the starting node to the queue. Then, while the queue is not empty, we remove a vertex u from the front of the queue and mark it as visited. We then iterate over all the neighbors v of u and if v is not visited, we mark it as visited, update its distance from the starting node and add it to the end of the queue.
Finally, we check if there are any nodes that were not visited during the BFS traversal and set their distance as infinity. We then return the distance list.
Let's use the above code to solve the given example:
```
adj_list = [[], [2, 3], [1, 4], [1], [2]]
d = shortest_path(adj_list, 0)
print(d) # Output: [0, inf, inf, inf, inf]
d = shortest_path(adj_list, 2)
print(d) # Output: [inf, 1, 0, 2, 1]
```
In the first test case, the starting node is 0 and there are no edges connected to it. Hence, the distance to all other nodes is infinity.
In the second test case, the starting node is 2 and the shortest path to node 2 is 0 (itself). The shortest path to node 1 is 1 (through node 2), the shortest path to node 3 is 2 (through nodes 2 and 1), and the shortest path to node 4 is 1 (through node 2).
阅读全文
相关推荐
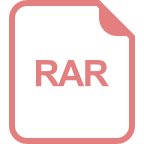
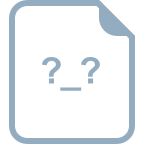
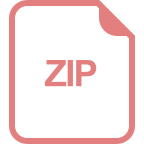
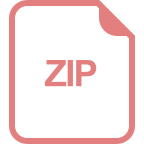
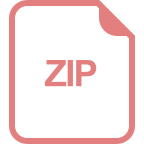
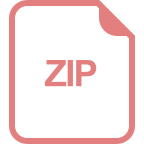
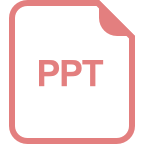
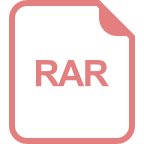
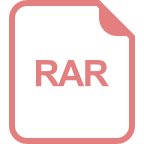





