请帮我写一个C# webforms前端使用bootstrap table加ajax调用一般处理程序,从Oracle数据库获取数据,显示一个包含图片的商品列表
时间: 2024-03-05 13:47:53 浏览: 22
好的,以下是一个示例代码:
前端代码(HTML/C# WebForms):
```html
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="WebApplication1.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<!-- 引入 Bootstrap 和 jQuery -->
<link href="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet" />
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/4.5.2/js/bootstrap.min.js"></script>
<!-- 引入 Bootstrap Table -->
<link href="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.18.3/bootstrap-table.min.css" rel="stylesheet" />
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.18.3/bootstrap-table.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/bootstrap-table/1.18.3/locale/bootstrap-table-zh-CN.min.js"></script>
<script type="text/javascript">
$(function () {
// 初始化表格
$('#table').bootstrapTable({
url: 'GetData.ashx', // 调用一般处理程序获取数据
method: 'post',
queryParams: function (params) {
return {
limit: params.limit, // 每页显示条数
offset: params.offset // 当前页码
}
},
pagination: true, // 开启分页
sidePagination: 'server', // 服务器端分页
pageNumber: 1, // 初始页码
pageSize: 10, // 初始每页显示条数
pageList: [10, 20, 50, 100], // 可选每页显示条数
columns: [
{
field: 'id',
title: '商品ID'
},
{
field: 'name',
title: '商品名称'
},
{
field: 'price',
title: '商品价格'
},
{
field: 'image',
title: '商品图片',
formatter: function (value, row, index) { // 自定义格式化函数
return '<img src="' + value + '" style="max-width: 100%; max-height: 100px;" />';
}
}
]
});
});
</script>
</head>
<body>
<table id="table"></table>
</body>
</html>
```
后端代码(C# 一般处理程序):
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Oracle.ManagedDataAccess.Client;
using Newtonsoft.Json;
namespace WebApplication1
{
/// <summary>
/// GetData 的摘要说明
/// </summary>
public class GetData : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
// 获取请求参数
int limit = int.Parse(context.Request.Form["limit"]); // 每页显示条数
int offset = int.Parse(context.Request.Form["offset"]); // 当前页码
// 连接 Oracle 数据库
string connStr = "Data Source=(DESCRIPTION=(ADDRESS_LIST=(ADDRESS=(PROTOCOL=TCP)(HOST=localhost)(PORT=1521)))(CONNECT_DATA=(SERVICE_NAME=ORCL)));User Id=scott;Password=tiger;";
using (OracleConnection conn = new OracleConnection(connStr))
{
conn.Open();
// 查询商品数据
string sql = "SELECT * FROM products ORDER BY id OFFSET :offset ROWS FETCH NEXT :limit ROWS ONLY";
using (OracleCommand cmd = new OracleCommand(sql, conn))
{
cmd.Parameters.Add(new OracleParameter(":offset", offset));
cmd.Parameters.Add(new OracleParameter(":limit", limit));
using (OracleDataReader reader = cmd.ExecuteReader())
{
// 将查询结果转换为 JSON 格式的字符串
List<Dictionary<string, object>> list = new List<Dictionary<string, object>>();
while (reader.Read())
{
Dictionary<string, object> dict = new Dictionary<string, object>();
dict.Add("id", reader.GetInt32(0));
dict.Add("name", reader.GetString(1));
dict.Add("price", reader.GetDecimal(2));
dict.Add("image", reader.GetString(3));
list.Add(dict);
}
string json = JsonConvert.SerializeObject(list);
// 返回 JSON 格式的字符串
context.Response.ContentType = "application/json";
context.Response.Write(json);
}
}
}
}
public bool IsReusable
{
get
{
return false;
}
}
}
}
```
说明:
1. 前端使用了 Bootstrap 和 jQuery,以及 Bootstrap Table 插件,其中 `#table` 是一个空的 `<table>` 元素,用于显示商品列表。
2. 前端通过 Ajax 调用一般处理程序 `GetData.ashx`,传递了 `limit` 和 `offset` 参数,表示每页显示条数和当前页码。
3. 一般处理程序 `GetData.ashx` 会连接 Oracle 数据库,查询商品数据,并将查询结果转换为 JSON 格式的字符串,最终返回给前端。
4. 前端通过 Bootstrap Table 插件,将 JSON 格式的字符串渲染成表格,并支持分页、排序等功能。其中 `columns` 属性指定了表格的列,其中包含一个自定义的 `formatter` 函数,用于将商品图片显示为图片元素。
相关推荐
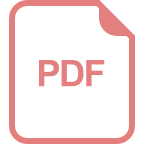
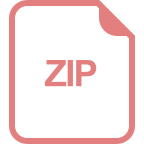
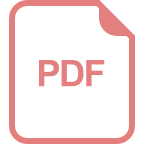
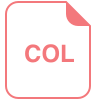
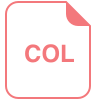
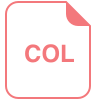
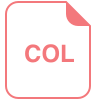
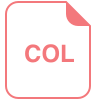









