cnn pytorch 绘制特征图
时间: 2023-06-17 13:06:46 浏览: 59
在 PyTorch 中,我们可以通过提取卷积神经网络 (CNN) 中每一层的特征图,来可视化模型的学习过程,了解模型在输入图像上的响应情况。下面是一个简单的实现步骤:
1. 加载训练好的 CNN 模型,并将其设置为 eval 模式。
```python
import torch
import torch.nn as nn
import torchvision.models as models
# 加载预训练模型
model = models.vgg16(pretrained=True)
# 设置为 eval 模式
model.eval()
```
2. 选择需要可视化的 CNN 层,并定义一个函数,用于提取该层特征图。
```python
# 选择需要可视化的层
layer = model.features[10]
# 定义函数,用于提取特定层的输出
def get_feature_map(model, layer, input):
# 创建一个新的网络,只包含指定层之前的部分
new_model = nn.Sequential(*list(model.children())[:layer+1])
# 提取指定层的输出
output = new_model(input)
return output
```
3. 加载一张图片,并将其输入模型,提取指定层的特征图。
```python
from PIL import Image
import torchvision.transforms as transforms
# 加载图片
image = Image.open('image.jpg')
# 对图片进行预处理
preprocess = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225])
])
input = preprocess(image).unsqueeze(0)
# 提取指定层的特征图
feature_map = get_feature_map(model, 10, input)
```
4. 将特征图可视化,可以通过 matplotlib 库实现。
```python
import matplotlib.pyplot as plt
# 将特征图转换为 numpy 数组
feature_map = feature_map.data.numpy()
# 可视化特征图
plt.imshow(feature_map[0, 0], cmap='gray')
plt.show()
```
以上就是一个简单的 CNN 特征图可视化的实现步骤。通过可视化特征图,我们可以更好地理解 CNN 模型的学习过程,以及模型对输入图像的响应情况。
相关推荐
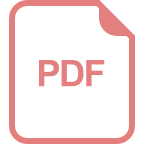
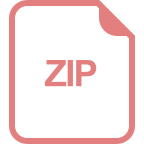














