解释这段代码:#include <stdio.h> #include <pthread.h> #include <unistd.h> void *fun(void *arg) { printf("I'm thread, Thread ID = %lu\n", pthread_self());//pthread_self函数,获取线程ID,其作用对应进程中 getpid() 函数。 return NULL; } int main(void) { pthread_t tid; pthread_create(&tid, NULL, fun, NULL); sleep(1); printf("I am main, my pid = %d\n", getpid()); return 0; }
时间: 2024-04-27 12:24:58 浏览: 86
这段代码使用了 pthread_create() 函数创建了一个新的线程,线程函数为 fun()。在 fun() 函数中,调用 pthread_self() 函数获取当前线程的 ID,并输出到标准输出中。在主函数中,调用 pthread_create() 函数创建线程,并传递线程函数 fun() 的地址作为参数。然后通过 sleep() 函数让主线程睡眠 1 秒钟,等待新创建的线程输出完毕。最后,主线程输出自己的进程 ID 到标准输出中,并结束程序。
总结来说,这段代码主要用于演示如何使用 pthread_create() 函数创建新的线程,以及如何使用 pthread_self() 函数获取当前线程的 ID。并通过输出线程的 ID 和主进程的 ID,来展示不同线程的执行顺序。其中,sleep() 函数用于控制主线程等待新创建的线程执行完毕。
相关问题
补全代码#include <stdio.h> #include <stdlib.h> #include <string.h> #include <errno.h> #include <unistd.h> #include <sys/types.h> #include <sys/socket.h> #include <sys/shm.h> #include <pthread.h> #include "mypthread.h" #include "serial.h" #include "zigbee.h" #include "my_camera.h" #include "server.h" static int zgbfd; static pthread_mutex_t cam_mutex; void *pthread_cam(void *arg) { } void *pthread_zgb(void *arg) { } void *pthread_cli(void *arg) { }
下面是对代码的补全:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/shm.h>
#include <pthread.h>
#include "mypthread.h"
#include "serial.h"
#include "zigbee.h"
#include "my_camera.h"
#include "server.h"
static int zgbfd;
static pthread_mutex_t cam_mutex;
void *pthread_cam(void *arg)
{
// 摄像头相关操作的具体实现
while (1) {
// 在这里实现摄像头的操作逻辑
// 例如获取摄像头图像并处理
// 使用互斥锁保护临界区
pthread_mutex_lock(&cam_mutex);
// 执行摄像头操作
pthread_mutex_unlock(&cam_mutex);
// 休眠一段时间
usleep(1000);
}
return NULL;
}
void *pthread_zgb(void *arg)
{
// Zigbee 相关操作的具体实现
while (1) {
// 在这里实现 Zigbee 的操作逻辑
// 例如发送或接收数据
// 使用互斥锁保护临界区
pthread_mutex_lock(&cam_mutex);
// 执行 Zigbee 操作
pthread_mutex_unlock(&cam_mutex);
// 休眠一段时间
usleep(1000);
}
return NULL;
}
void *pthread_cli(void *arg)
{
// 客户端连接和通信操作的具体实现
int client_sock = *((int*)arg);
char buffer[1024];
while (1) {
// 在这里实现客户端连接和通信的操作逻辑
// 例如接收客户端消息并处理
memset(buffer, 0, sizeof(buffer));
int ret = read(client_sock, buffer, sizeof(buffer));
if (ret <= 0) {
// 客户端断开连接或出错,结束线程
close(client_sock);
break;
}
// 处理客户端消息
}
return NULL;
}
```
以上补全了 `pthread_cam`、`pthread_zgb` 和 `pthread_cli` 函数的代码实现部分。你可以根据具体需求在这些函数中添加逻辑来完成相应的操作。注意使用互斥锁来保护临界区,以避免多个线程同时访问造成的竞争条件。
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <errno.h> #include <unistd.h> #include <sys/types.h> #include <sys/socket.h> #include <sys/shm.h> #include <pthread.h> #include "mypthread.h" #include "serial.h" #include "zigbee.h" #include "my_camera.h" #include "server.h" static int zgbfd; static pthread_mutex_t cam_mutex; void *pthread_cam(void *arg) { } void *pthread_zgb(void *arg) { } void *pthread_cli(void *arg) { }
这段代码是一个多线程服务器程序。它使用了一些标准库和系统库的头文件,并定义了一些全局变量和线程函数。
- `zgbfd` 是一个表示 Zigbee 连接的文件描述符。
- `cam_mutex` 是一个互斥锁,用于保护摄像头操作的临界区。
然后,代码定义了三个线程函数:`pthread_cam`、`pthread_zgb`和`pthread_cli`。这些函数将在多线程环境下执行不同的任务。
`pthread_cam` 函数用于处理摄像头相关的操作,具体实现需要补充在函数体内。
`pthread_zgb` 函数用于处理 Zigbee 相关的操作,具体实现需要补充在函数体内。
`pthread_cli` 函数用于处理客户端连接和通信的操作,具体实现需要补充在函数体内。
这只是一个代码框架,具体的实现可能需要根据具体需求进行修改和补充。
阅读全文
相关推荐



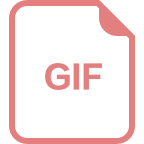
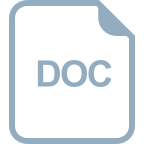








