QDBUS 热插拔U盘检测
时间: 2023-09-03 11:07:37 浏览: 66
在DBus中,可以使用Udev规则监视USB设备的插拔事件。Udev是Linux内核中的一个子系统,用于管理系统设备节点。DBus可以与Udev集成,从而能够实现对USB设备插拔事件的监视。
下面是一个简单的利用DBus和Udev实现热插拔U盘检测的示例程序:
```cpp
#include <QtCore/QCoreApplication>
#include <QtDBus/QtDBus>
#include <QDebug>
QString deviceNodeFromUdev(QString devPath)
{
QDBusInterface udev("org.freedesktop.UDisks2", devPath, "org.freedesktop.UDisks2.Block", QDBusConnection::systemBus());
if (udev.isValid()) {
QDBusReply<QString> deviceNode = udev.call("Device");
if (deviceNode.isValid()) {
return deviceNode.value();
}
}
return QString();
}
void deviceAdded(QString devPath)
{
QString deviceNode = deviceNodeFromUdev(devPath);
if (!deviceNode.isEmpty()) {
qDebug() << "Device added:" << deviceNode;
}
}
void deviceRemoved(QString devPath)
{
QString deviceNode = deviceNodeFromUdev(devPath);
if (!deviceNode.isEmpty()) {
qDebug() << "Device removed:" << deviceNode;
}
}
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
QDBusConnection systemBus = QDBusConnection::systemBus();
if (!systemBus.isConnected()) {
qCritical() << "Cannot connect to the D-Bus system bus.";
return 1;
}
if (!systemBus.registerService("org.example.UdevExample")) {
qCritical() << "Cannot register D-Bus service.";
return 1;
}
if (!systemBus.registerObject("/org/example/UdevExample", &app)) {
qCritical() << "Cannot register D-Bus object.";
return 1;
}
QDBusInterface udev("org.freedesktop.UDisks2", "/org/freedesktop/UDisks2", "org.freedesktop.DBus.ObjectManager", systemBus);
if (!udev.isValid()) {
qCritical() << "Cannot get UDisks2 object manager interface.";
return 1;
}
QDBusReply<QDBusObjectPath> reply = udev.call("GetManagedObjects");
if (!reply.isValid()) {
qCritical() << "Cannot get UDisks2 managed objects.";
return 1;
}
QDBusArgument arg = reply.value().toVariant().value<QDBusArgument>();
arg.beginArray();
while (!arg.atEnd()) {
QString objPath;
QDBusArgument objArg;
arg.beginStructure();
arg >> objPath >> objArg;
arg.endStructure();
if (objPath.startsWith("/org/freedesktop/UDisks2/block_devices/")) {
QDBusInterface udevInterface("org.freedesktop.UDisks2", objPath, "org.freedesktop.DBus.Properties", systemBus);
if (udevInterface.isValid()) {
QDBusReply<QVariantMap> reply = udevInterface.call("GetAll", "org.freedesktop.UDisks2.Block");
if (reply.isValid()) {
QVariantMap properties = reply.value();
if (properties.contains("DeviceFile")) {
QString deviceNode = properties["DeviceFile"].toString();
qDebug() << "Initial device:" << deviceNode;
}
}
}
systemBus.connect("org.freedesktop.UDisks2", objPath, "org.freedesktop.DBus.Properties", "PropertiesChanged", &app, SLOT(deviceAdded(QString,QString,QVariantMap)));
}
arg.next();
}
arg.endArray();
return app.exec();
}
```
该程序首先连接DBus系统总线,并注册了一个名为"org.example.UdevExample"的DBus服务和一个DBus对象"/org/example/UdevExample"。然后,程序获取UDisks2对象管理器的接口,并调用"GetManagedObjects"方法获取当前已经连接到系统中的所有UDisks2对象及其属性。
程序遍历所有UDisks2对象,并对每个块设备对象注册了一个DBus信号接收器,用来监听该块设备对象的属性变化事件。当有U盘插入或拔出时,程序将自动检测到该事件,并输出U盘设备节点的路径。
需要注意的是,该程序需要在root权限下运行,否则无法获取Udev和DBus的权限。
相关推荐
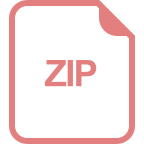














