xlsx文件转pdf文件
时间: 2023-11-24 07:08:22 浏览: 199
你可以使用Python中的openpyxl和reportlab库来将xlsx文件转换为pdf文件。下面是一些示例代码:
```python
from openpyxl import load_workbook
from reportlab.pdfgen import canvas
# Load the Excel file
workbook = load_workbook(filename='example.xlsx')
worksheet = workbook.active
# Create a new PDF file
pdf = canvas.Canvas('example.pdf')
# Loop through the rows and columns of the Excel file, and write the values to the PDF
for row in worksheet.iter_rows(values_only=True):
for col_index, cell_value in enumerate(row):
pdf.drawString(col_index * 100, row_index * 20, str(cell_value))
# Save the PDF file
pdf.save()
```
这段代码将Excel文件中的内容逐行逐列地写入PDF文件中。你需要根据自己的需求来修改代码。记得安装openpyxl和reportlab库。
相关问题
java 写xlsx文件转pdf文件
你可以使用 Apache POI 库来读写 Excel 文件,使用 iText 库来生成 PDF 文件。下面是一个简单的示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import com.itextpdf.text.Document;
import com.itextpdf.text.pdf.PdfWriter;
import com.itextpdf.text.pdf.PdfPTable;
import com.itextpdf.text.pdf.PdfPCell;
import java.io.FileOutputStream;
import java.io.FileInputStream;
import java.io.File;
public class ExcelToPdfConverter {
public static void main(String[] args) {
String excelFilePath = "path/to/excel/file.xlsx";
String pdfFilePath = "path/to/pdf/file.pdf";
try {
FileInputStream inputStream = new FileInputStream(new File(excelFilePath));
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0);
Document document = new Document();
PdfWriter.getInstance(document, new FileOutputStream(pdfFilePath));
document.open();
PdfPTable table = new PdfPTable(sheet.getRow(0).getLastCellNum());
for (Row row : sheet) {
for (Cell cell : row) {
table.addCell(cell.toString());
}
}
document.add(table);
document.close();
workbook.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
上面的代码将 Excel 文件的第一个 sheet 转换成了 PDF 文件。你需要将 `path/to/excel/file.xlsx` 和 `path/to/pdf/file.pdf` 替换成实际的文件路径。
编写一个js方法,前端将xlsx文件转换成pdf文件
在JavaScript中,将XLSX文件转换为PDF文件通常需要借助于第三方库,因为浏览器本身并不支持直接处理这两种文件格式之间的转换。一个常用的选择是`xlsx`库用于读取Excel文件,然后结合`html2canvas`或`jsPDF`等工具将其转换为PDF。
以下是一个简单的示例,使用`xlsx`和`jspdf`库来完成这个过程:
```javascript
// 引入必要的库
const XLSX = require('xlsx');
const jsPDF = require('jspdf');
async function xlsxToPdf(xlsxData, fileName) {
// 首先,加载并解析XLSX文件
const workbook = XLSX.read(xlsxData, { type: 'buffer' });
// 获取第一个工作表
const sheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[sheetName];
// 创建一个新的PDF文档
const pdf = new jsPDF();
// 将HTML内容添加到PDF
let html = '<table>';
for (let row of XLSX.utils.sheet_to_json(worksheet)) {
html += '<tr>';
Object.values(row).forEach(value => {
html += `<td>${value}</td>`;
});
html += '</tr>';
}
html += '</table>';
// 添加HTML到PDF
pdf.html(html);
// 保存PDF文件
pdf.save(fileName + '.pdf');
}
// 使用方法
let xlsxFileBuffer; // 假设已经获取到了xlsx文件的Buffer数据
xlsxToPdf(xlsxFileBuffer, 'output');
```
注意:这只是一个基础示例,并未包含错误处理和优化处理。实际应用中,你可能需要根据实际的XLSX表格结构调整HTML生成的方式,或者考虑使用更专业的库如`xlsx-to-pdf`来进行转换。
阅读全文
相关推荐
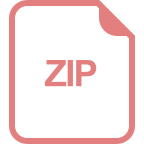
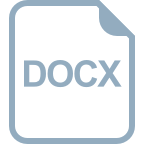
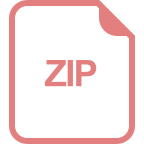
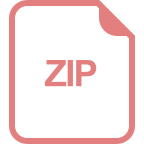
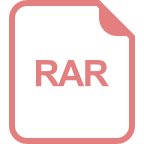











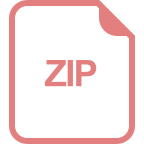