java统计员工人数、平均工资、工资总额、最高工资、统计工资的所有信息。 counting() 、averagingDouble()、maxBy()、summingInt()、summarizingDouble()的代码
时间: 2023-06-09 19:08:33 浏览: 133
好的,您的问题是如何使用Java统计员工工资信息。以下是一种实现方式:
```
import java.util.*;
import java.util.stream.Collectors;
public class EmployeeStatistics {
public static void main(String[] args) {
// Create a list of employees
List<Employee> employees = Arrays.asList(
new Employee("Alice", 50000),
new Employee("Bob", 60000),
new Employee("Charlie", 70000),
new Employee("David", 55000),
new Employee("Eva", 65000)
);
// Count the number of employees
long count = employees.stream().count();
System.out.println("Number of employees: " + count);
// Calculate the average salary of employees
double averageSalary = employees.stream().collect(Collectors.averagingDouble(Employee::getSalary));
System.out.println("Average salary: " + averageSalary);
// Sum up the total salary of employees
double totalSalary = employees.stream().collect(Collectors.summingDouble(Employee::getSalary));
System.out.println("Total salary: " + totalSalary);
// Find the employee with the highest salary
Optional<Employee> maxSalaryEmployee = employees.stream().collect(Collectors.maxBy(Comparator.comparingDouble(Employee::getSalary)));
if (maxSalaryEmployee.isPresent()) {
System.out.println("Employee with highest salary: " + maxSalaryEmployee.get().getName() + " (Salary: " + maxSalaryEmployee.get().getSalary() + ")");
}
// Get a summary of the employee salaries
DoubleSummaryStatistics salaryStatistics = employees.stream().collect(Collectors.summarizingDouble(Employee::getSalary));
System.out.println("Salary Statistics: " + salaryStatistics);
}
}
class Employee {
private String name;
private double salary;
public Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
public String getName() {
return name;
}
public double getSalary() {
return salary;
}
}
```
在这个例子中,我们使用了Java 8的流式API来对员工信息进行统计。首先,我们创建了一个Employee类来表示员工,其中包含了员工的姓名和薪资信息。然后,我们创建了一个包含了员工对象的列表。接下来,我们使用Java 8的流式API对这个列表进行统计分析。
我们使用count()方法来获取员工总数。我们使用averagingDouble()方法来计算员工的平均工资。我们使用summingInt()方法来计算员工的工资总额。我们使用maxBy()方法和Comparator.comparingDouble()方法来找到薪资最高的员工。最后,我们使用summarizingDouble()方法来获得员工薪资的所有统计信息。
以上就是一个简单的Java代码例子,用于统计员工工资信息。注意:这里的实现方式仅为参考之用,具体实现方式因应用场景而异。
相关推荐
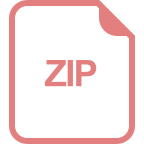
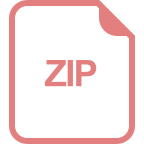















