vue复制图片到剪切板兼容edge浏览器
时间: 2024-09-07 17:01:17 浏览: 85
在Vue.js中,复制图片到剪贴板通常通过`clipboard.js`库来实现,这是一个轻量级的JavaScript库,用于在现代浏览器上提供跨平台的剪贴板功能。对于Edge浏览器的支持,`clipboard.js`已经做得很好。
首先,你需要安装`clipboard.js`:
```bash
npm install clipboardjs
# 或者
yarn add clipboardjs
```
然后,在你的Vue组件中引入并使用它:
```html
<!-- 引入script标签 -->
<script src="path/to/clipboard.min.js"></script>
<!-- 使用ref绑定到img元素 -->
<template>
<div>
<img ref="imageToCopy" :src="yourImageSrc" />
<button @click="copyImage">复制图片</button>
</div>
</template>
<script>
import ClipboardJS from 'clipboard';
export default {
data() {
return {
yourImageSrc: 'your-image-url', // 图片URL
};
},
mounted() {
this.clipboard = new ClipboardJS('.copy-button'); // 将按钮绑定到ClipboardJS实例
},
methods: {
copyImage() {
if (this.$refs.imageToCopy) { // 确保图片元素存在
const imageDataUrl = this.$refs.imageToCopy.src; // 获取图片数据URL
this.clipboard.writeText(imageDataUrl); // 调用复制操作
}
},
},
};
</script>
```
这里假设你有一个`.copy-button`的CSS选择器指向你的复制按钮。如果你需要处理Edge浏览器特有的兼容性问题,可以在`mounted`生命周期钩子里添加条件判断检查是否支持`writeText`方法:
```javascript
methods: {
copyImage() {
if (typeof this.clipboard === 'undefined' || !this.clipboard.writeText) {
// Edge浏览器可能不支持,可以考虑使用其他方法如toDataURL
const canvas = document.createElement('canvas');
canvas.width = this.$refs.imageToCopy.naturalWidth;
canvas.height = this.$refs.imageToCopy.naturalHeight;
canvas.getContext('2d').drawImage(this.$refs.imageToCopy, 0, 0);
const imageDataUrl = canvas.toDataURL('image/png');
navigator.clipboard.writeText(imageDataUrl); // 写入剪贴板
} else {
this.clipboard.writeText(this.$refs.imageToCopy.src);
}
},
},
```
阅读全文
相关推荐




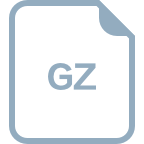




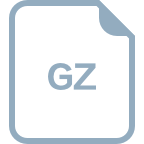

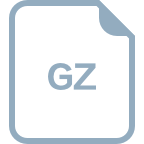

