用C语言完成代码实现:结合线性结构特点,将某班级的学生成绩单设计为线性表,表中每位学生的信息包括学号、姓名、成绩,设计算法统计及格人数和最高分。
时间: 2024-10-28 20:08:05 浏览: 11
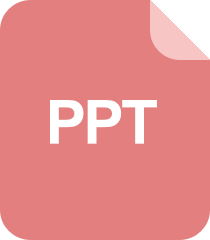
数据结构(C语言版):第1章 绪论.ppt
在C语言中,我们可以创建一个结构体来存储学生的学号、姓名和成绩,然后使用数组或链表作为线性表来存储所有学生的信息。这里我们选择动态分配内存的链表来实现,因为这样可以方便地添加或删除学生。以下是基本的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生信息结构体
typedef struct {
int student_id; // 学号
char name[50]; // 姓名
float score; // 成绩
} Student;
// 简单的学生链表节点结构
typedef struct Node {
Student info;
struct Node* next;
} Node;
// 动态创建并初始化新节点
Node* createStudent(int id, const char* name, float score) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode) {
newNode->info.student_id = id;
strncpy(newNode->info.name, name, sizeof(newNode->info.name) - 1);
newNode->info.score = score;
newNode->next = NULL;
}
return newNode;
}
// 链表插入操作
void insertStudent(Node** head, int id, const char* name, float score) {
Node* newNode = createStudent(id, name, score);
if (*head == NULL) {
*head = newNode;
} else {
Node* curr = *head;
while (curr->next != NULL) {
curr = curr->next;
}
curr->next = newNode;
}
}
// 统计及格人数(通常设定60分为及格线)
int countPassingStudents(Node* head) {
int passingCount = 0;
Node* curr = head;
while (curr != NULL) {
if (curr->info.score >= 60) {
passingCount++;
}
curr = curr->next;
}
return passingCount;
}
// 查找最高分
float findHighestScore(Node* head) {
Node* curr = head;
float highestScore = 0;
while (curr != NULL) {
if (curr->info.score > highestScore) {
highestScore = curr->info.score;
}
curr = curr->next;
}
return highestScore;
}
int main() {
// 初始化链表为空
Node* studentsHead = NULL;
// 添加学生到链表
insertStudent(&studentsHead, 1, "张三", 85.0);
insertStudent(&studentsHead, 2, "李四", 70.0);
insertStudent(&studentsHead, 3, "王五", 92.0);
// ... 更多学生
// 统计及格人数和最高分
int passingStudents = countPassingStudents(studentsHead);
float highestScore = findHighestScore(studentsHead);
printf("及格人数: %d\n", passingStudents);
printf("最高分: %.2f\n", highestScore);
return 0;
}
```
在这个例子中,`main()`函数负责创建并管理链表,并通过`countPassingStudents()`和`findHighestScore()`函数计算及格人数和最高分。
阅读全文
相关推荐
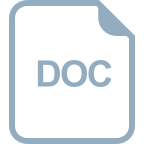
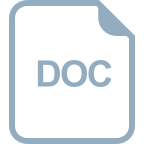

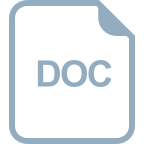
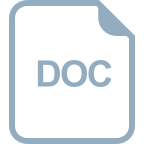
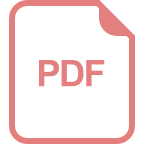
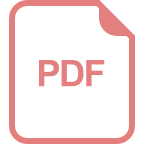
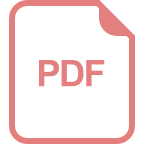
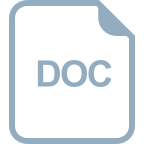
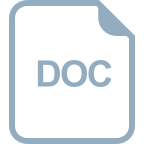
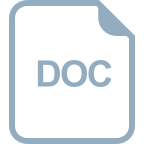
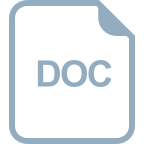
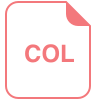