用C++代码实现置换密码
时间: 2023-10-24 08:05:57 浏览: 199
以下是一个简单的C代码实现置换密码的例子:
```
#include <stdio.h>
#include <string.h>
// 定义置换表
const int table[8] = {2, 4, 1, 6, 3, 8, 5, 7};
// 置换函数
void substitution(char *str) {
int len = strlen(str);
char temp[len];
for (int i = 0; i < len; i++) {
temp[table[i % 8] - 1] = str[i];
}
temp[len] = '\0';
strcpy(str, temp);
}
int main() {
char str[100];
printf("请输入明文:");
scanf("%s", str);
substitution(str);
printf("密文为:%s\n", str);
return 0;
}
```
这个例子中,我们定义了一个置换表,然后编写了一个置换函数,用于将明文中的字符按照置换表进行置换,生成密文。最后,在main函数中,我们读入明文,调用置换函数生成密文,并输出结果。
当然,这只是一个简单的例子,实际使用中需要根据具体情况进行修改和完善。
相关问题
应用密码学 古典密码中的置换密码 C++代码实现
应用密码学是一门研究如何使用数学原理来保护信息安全的学科,它涉及加密、解密、数据完整性验证以及身份认证等多种技术。其中,置换密码(Caesar Cipher)是一种最古老的加密方法之一,它通过简单的字母替换规则来隐藏信息。
在古典密码学中的置换密码,比如凯撒密码(Caesar Cipher),最常见的做法是将字母表中的每个字符按照固定的位移量向后移动。例如,凯撒密码的基本思想是将字母表中的每一个字母向前移动固定数位,如移动3位,A就变成D,B变E,以此类推。
下面是一个简单的C++代码实现凯撒密码的加密过程:
```cpp
#include <iostream>
#include <string>
std::string caesar_cipher_encrypt(std::string plaintext, int shift) {
std::string ciphertext = "";
for (char c : plaintext) {
if (isalpha(c)) { // 检查是否为字母
char encrypted_char;
if (islower(c))
encrypted_char = static_cast<char>((c + shift - 'a') % 26 + 'a');
else
encrypted_char = static_cast<char>((c + shift - 'A') % 26 + 'A');
ciphertext += encrypted_char;
} else {
ciphertext += c; // 非字母字符直接保留
}
}
return ciphertext;
}
int main() {
std::string input = "Hello, World!";
int shift = 3;
std::string encrypted = caesar_cipher_encrypt(input, shift);
std::cout << "Original text: " << input << "\n";
std::cout << "Encrypted text: " << encrypted << "\n";
return 0;
}
```
在这个例子中,`caesar_cipher_encrypt`函数接受明文和位移量作为参数,然后对输入字符串中的每个字符进行加密处理。非字母字符保持不变。
c++ 置换密码代码
C语言中的置换密码代码是一种简单的密码加密方法,它通过将明文中的每个字符按照一定规则进行替换,从而得到密文。我们先定义一个置换表,将每个字母对应到另一个字母或者符号上,然后遍历明文,将每个字符根据置换表进行替换,得到密文。
代码示例:
```c
#include <stdio.h>
#include <string.h>
// 定义置换表
char substitution_table[26] = {
'x', 'y', 'z', 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w'
};
// 置换密码加密函数
void substitution_cipher(char *plaintext, char *ciphertext) {
int length = strlen(plaintext);
for (int i = 0; i < length; i++) {
if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
ciphertext[i] = substitution_table[plaintext[i] - 'a'];
} else {
ciphertext[i] = plaintext[i];
}
}
}
int main() {
char plaintext[100];
char ciphertext[100];
printf("请输入明文:");
scanf("%s", plaintext);
substitution_cipher(plaintext, ciphertext);
printf("密文为:%s\n", ciphertext);
return 0;
}
```
这段代码实现了简单的置换密码加密,通过输入明文后,就可以得到对应的密文。当然,这种加密方法非常简单,只是用来演示加密原理,实际使用中需要更加复杂的加密算法来保障安全。
阅读全文
相关推荐
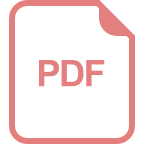
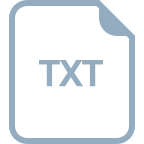
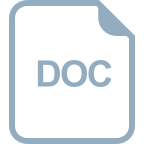
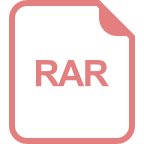
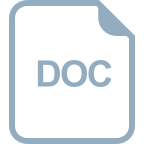
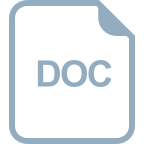
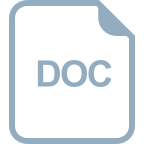


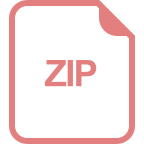
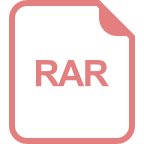
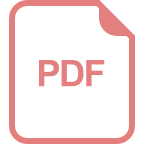

