use java Return the number of even ints in the given array. Note: the % "mod" operator computes the remainder, e.g. 5 % 2 is 1. countEvens([2, 1, 2, 3, 4]) → 3 countEvens([2, 2, 0]) → 3 countEvens([1, 3, 5]) → 0
时间: 2023-11-26 19:02:59 浏览: 16
可以使用以下代码来实现:
```java
public int countEvens(int[] nums) {
int count = 0;
for (int i = 0; i < nums.length; i++) {
if (nums[i] % 2 == 0) {
count++;
}
}
return count;
}
```
该方法遍历整个数组,如果当前数字是偶数,则将计数器加1。最后返回计数器的值即为偶数个数。
相关问题
Return the number of even ints in the given array. Note: the % "mod" operator computes the remainder, e.g. 5 % 2 is 1.
Sure, I can help you with that! Here's a Python code snippet that counts the number of even integers in an array:
```python
def count_even_numbers(arr):
count = 0
for num in arr:
if num % 2 == 0:
count += 1
return count
```
You can call this function with your array as an argument to get the number of even integers in the array. For example:
```python
arr = [1, 2, 3, 4, 5, 6]
count = count_even_numbers(arr)
print(count) # Output: 3
```
In this example, `count` will be equal to 3 because there are three even integers in the array (`2`, `4`, and `6`).
The number of columns in Aeq must be the same as the number of elements of f.
This error message typically occurs when using the MATLAB function `linprog` for linear programming problems. The message means that the number of columns in the matrix `Aeq` (which represents the equality constraints) must be equal to the number of elements in the vector `f` (which represents the objective function coefficients).
To resolve this error, you need to check that the dimensions of `Aeq` and `f` are compatible. Specifically, the number of columns in `Aeq` should be the same as the length of `f`. If they are not the same, you need to adjust the dimensions of one or both of these inputs so that they are compatible.
One common mistake that can cause this error is forgetting to transpose `f` when using row vectors instead of column vectors. In this case, you can fix the error by transposing `f` using the apostrophe operator, like this: `linprog(f', [], [], Aeq, beq, lb, ub)`.
相关推荐
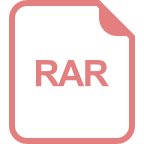
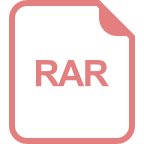
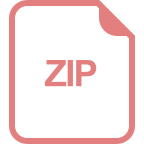













