#include <stdio.h> void sort(int *a); int main() { int num[7],i; for(i=0; i<7; i++) scanf("%d",&num[i]); sort(num); return 0; }
时间: 2024-01-31 19:03:23 浏览: 12
这段代码的作用是读入7个整数,然后调用`sort`函数对它们进行排序。`sort`函数的声明已经给出,应该是一个对整型指针进行操作的函数。因此,`sort`函数的定义应该是这样的:
```c
void sort(int *a) {
// 对a所指向的数组进行排序
}
```
您可以在这个函数中使用任何排序算法来实现对数组的排序。常见的排序算法有冒泡排序、插入排序、选择排序、快速排序等。
相关问题
补齐以下代码#include <stdio.h> #include <stdlib.h> struct node { int data; struct node *next; }; void sort(struct node *head); //采用选择排序法思想,对结点数据进行交换处理 void destroy(struct node* head); struct node* creatlink(); void traverse(struct node* head); int m
代码中缺少函数 `main`,需要补充。
以下是完整代码:
```
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
void sort(struct node *head);
void destroy(struct node* head);
struct node* creatlink();
void traverse(struct node* head);
int main() {
struct node *head = creatlink(); // 创建带头结点的链表
sort(head); // 对链表进行排序
traverse(head); // 遍历链表
destroy(head); // 销毁链表
return 0;
}
struct node *creatlink() {
struct node *head = (struct node*)malloc(sizeof(struct node)); // 创建头结点
struct node *p = head;
int num;
printf("请输入数据(输入0结束):\n");
scanf("%d", &num);
while (num) {
struct node *newnode = (struct node*)malloc(sizeof(struct node));
newnode->data = num;
newnode->next = NULL;
p->next = newnode;
p = p->next;
scanf("%d", &num);
}
return head;
}
void sort(struct node *head) {
struct node *p = head->next;
while (p != NULL) {
struct node *q = p->next;
while (q != NULL) {
if (p->data > q->data) {
int temp = p->data;
p->data = q->data;
q->data = temp;
}
q = q->next;
}
p = p->next;
}
}
void traverse(struct node *head) {
struct node *p = head->next;
printf("排序后的链表数据为:\n");
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
void destroy(struct node *head) {
struct node *p = head;
while (p != NULL) {
struct node *q = p->next;
free(p);
p = q;
}
}
```
#include<stdio.h> #include<string.h> typedef struct date { int year; int month; int day; }DATE; typedef struct student { int num; char name[20]; char sex; DATE birthday; float score; }STUDENT; void input(STUDENT *s); void output(STUDENT s); /********** Begin **********/ /********** End **********/
完整代码如下所示:
```c
#include <stdio.h>
#include <string.h>
typedef struct date {
int year;
int month;
int day;
} DATE;
typedef struct student {
int num;
char name[20];
char sex;
DATE birthday;
float score;
} STUDENT;
void input(STUDENT *s);
void output(STUDENT s);
void sort(STUDENT s[], int n);
int main() {
STUDENT s[5];
int i;
for (i = 0; i < 5; i++) {
printf("请输入第%d个学生的信息:\n", i + 1);
input(&s[i]);
}
sort(s, 5);
printf("\n排序后的学生信息为:\n");
for (i = 0; i < 5; i++) {
output(s[i]);
}
return 0;
}
void input(STUDENT *s) {
printf("学号:");
scanf("%d", &s -> num);
printf("姓名:");
scanf("%s", s -> name);
printf("性别:");
scanf(" %c", &s -> sex);
printf("生日:");
scanf("%d/%d/%d", &s -> birthday.year, &s -> birthday.month, &s -> birthday.day);
printf("成绩:");
scanf("%f", &s -> score);
}
void output(STUDENT s) {
printf("学号:%d,姓名:%s,性别:%c,生日:%d/%d/%d,成绩:%.2f\n",
s.num, s.name, s.sex, s.birthday.year, s.birthday.month, s.birthday.day, s.score);
}
void sort(STUDENT s[], int n) {
int i, j;
STUDENT temp;
for (i = 0; i < n - 1; i++) {
for (j = i + 1; j < n; j++) {
if (s[i].score < s[j].score) {
temp = s[i];
s[i] = s[j];
s[j] = temp;
}
}
}
}
```
这段代码中,定义了一个main函数,用于程序的入口,创建了一个学生信息数组s。在main函数中,使用for循环对每个学生的信息进行输入。输入完成后,调用sort函数对学生信息数组s进行排序。最后,使用for循环对排序后的学生信息进行输出。
阅读全文
相关推荐
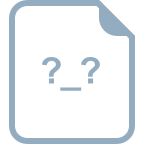
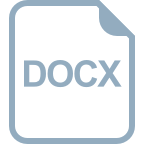










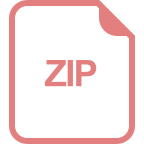
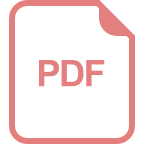
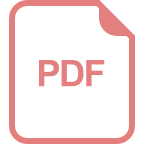