单元测试HttpServletRequest如何引入
时间: 2023-11-25 08:42:20 浏览: 35
要在单元测试中引入HttpServletRequest对象,可以使用MockHttpServletRequest类。您可以在测试方法中创建一个MockHttpServletRequest对象,并使用setMethod、setRequestURI、addParameter等方法设置相应的属性和参数。然后,您可以在测试中使用这个MockHttpServletRequest对象。
示例代码如下:
import org.springframework.mock.web.MockHttpServletRequest;
...
@Test
public void testMethod() {
MockHttpServletRequest request = new MockHttpServletRequest();
request.setMethod("GET");
request.setRequestURI("/mock");
request.addParameter("name", "MockMvc");
// 在这里使用MockHttpServletRequest对象进行测试
}
相关问题
单元测试HttpServletRequest req = attributes.getRequest()
在进行单元测试时,可以使用MockHttpServletRequest来模拟HttpServletRequest对象。MockHttpServletRequest是Spring Framework提供的一个工具类,用于模拟请求对象。使用该类可以方便地创建HttpServletRequest对象并设置相应的属性和参数。
以下是一个示例代码:
```
MockHttpServletRequest req = new MockHttpServletRequest();
req.setMethod("GET");
req.setRequestURI("/example");
req.addParameter("param1", "value1");
```
在这个例子中,首先创建了一个MockHttpServletRequest对象,然后设置了请求的方法为GET,请求的URI为/example,并添加了一个名为param1的参数,值为value1。
通过这样的方式,你可以在单元测试中模拟HttpServletRequest对象的各种属性和参数,以便进行相关的测试。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [Spring容器托管的HttpServletRequest对象被获取之后再次获取](https://blog.csdn.net/boonya/article/details/88551832)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [response.sendRedirect()与request.getRequestDispatcher().forward()区别](https://blog.csdn.net/qq_34471305/article/details/60462838)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
单元测试模拟一个httpservletrequest
可以使用Mockito框架来模拟一个HttpServletRequest对象,下面是一个示例代码:
```java
import static org.mockito.Mockito.*;
import javax.servlet.http.*;
import org.junit.Test;
public class MyServletTest {
@Test
public void testDoPost() throws Exception {
HttpServletRequest request = mock(HttpServletRequest.class);
HttpServletResponse response = mock(HttpServletResponse.class);
when(request.getParameter("name")).thenReturn("John");
when(request.getParameter("age")).thenReturn("30");
new MyServlet().doPost(request, response);
verify(request, atLeast(1)).getParameter("name");
verify(request, atLeast(1)).getParameter("age");
verify(response, atLeast(1)).getWriter();
// add more assertions as needed
}
}
```
在上面的示例中,我们使用Mockito框架创建了一个HttpServletRequest和HttpServletResponse的模拟对象,并使用when()方法设置了模拟HttpServletRequest对象的getParameter()方法返回值。然后,我们调用了MyServlet类的doPost()方法,并使用verify()方法来验证模拟对象的方法是否被正确调用。
相关推荐
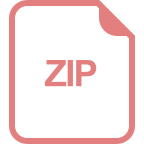
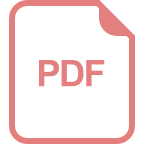






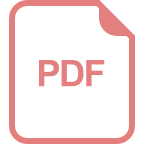
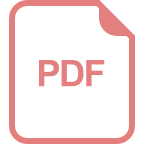
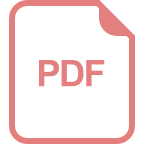
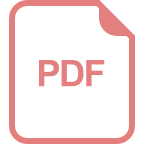
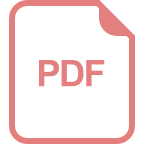
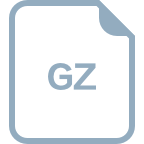