应用标准 C++ 模板库(STL)通用算法和函数对象实现查找与排序。
时间: 2024-03-10 07:48:55 浏览: 82
标准 C++ 模板库(STL)提供了丰富的通用算法和函数对象,可以方便地实现查找和排序操作。
1. 查找操作
STL 中提供了多种查找算法,如 find、find_if、count、binary_search、lower_bound、upper_bound 等。
以 find 算法为例,用法如下:
```c++
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec{1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Found 3 at position " << std::distance(vec.begin(), it) << std::endl;
} else {
std::cout << "3 not found" << std::endl;
}
return 0;
}
```
2. 排序操作
STL 中提供了多种排序算法,如 sort、stable_sort、partial_sort 等。
以 sort 算法为例,用法如下:
```c++
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec{3, 2, 1, 4, 5};
std::sort(vec.begin(), vec.end());
for (int i : vec) {
std::cout << i << " ";
}
std::cout << std::endl;
return 0;
}
```
以上是使用 STL 实现查找和排序操作的简单示例。在实际使用中,可以根据需要选择适合的算法和函数对象,并结合具体的数据结构和业务需求进行使用。
阅读全文
相关推荐
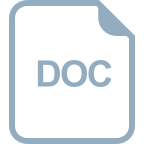
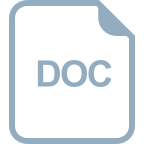
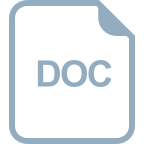
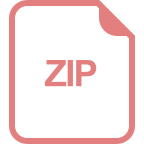
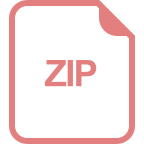
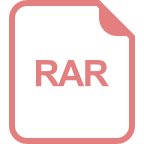
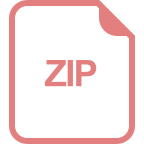
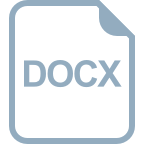
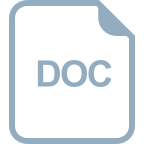
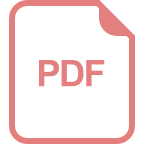
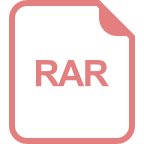
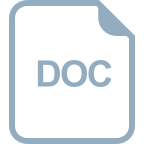
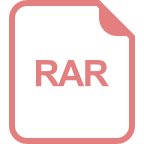
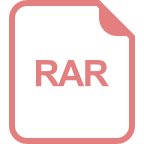
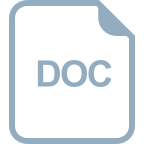
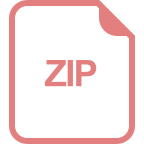
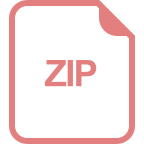
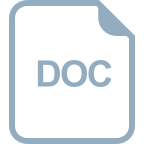
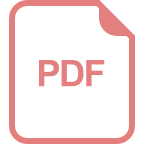