java制作图书馆里系统
时间: 2023-10-01 21:05:40 浏览: 37
要制作一个图书馆管理系统,需要使用Java中的GUI库来实现用户界面,同时需要使用数据库来存储图书和读者的信息。以下是一个简单的示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.sql.*;
public class LibrarySystem extends JFrame implements ActionListener {
private JLabel label1, label2, label3, label4, label5;
private JTextField textField1, textField2, textField3, textField4, textField5;
private JButton button1, button2, button3, button4;
private JTable table;
private JScrollPane scrollPane;
private Connection conn;
public LibrarySystem() {
// 创建界面组件
label1 = new JLabel("图书编号");
label2 = new JLabel("图书名称");
label3 = new JLabel("作者");
label4 = new JLabel("出版社");
label5 = new JLabel("读者编号");
textField1 = new JTextField();
textField2 = new JTextField();
textField3 = new JTextField();
textField4 = new JTextField();
textField5 = new JTextField();
button1 = new JButton("添加");
button2 = new JButton("删除");
button3 = new JButton("更新");
button4 = new JButton("查询");
table = new JTable();
scrollPane = new JScrollPane(table);
// 创建数据库连接
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/library", "root", "password");
} catch (Exception ex) {
ex.printStackTrace();
}
// 添加组件到窗口中
JPanel panel = new JPanel(new GridLayout(6, 2));
panel.add(label1);
panel.add(textField1);
panel.add(label2);
panel.add(textField2);
panel.add(label3);
panel.add(textField3);
panel.add(label4);
panel.add(textField4);
panel.add(label5);
panel.add(textField5);
panel.add(button1);
panel.add(button2);
panel.add(button3);
panel.add(button4);
add(panel, BorderLayout.NORTH);
add(scrollPane, BorderLayout.CENTER);
// 设置窗口属性
setTitle("图书馆管理系统");
setSize(800, 600);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
// 添加事件监听器
button1.addActionListener(this);
button2.addActionListener(this);
button3.addActionListener(this);
button4.addActionListener(this);
}
@Override
public void actionPerformed(ActionEvent e) {
// 处理添加按钮事件
if (e.getSource() == button1) {
try {
PreparedStatement ps = conn.prepareStatement("INSERT INTO books VALUES (?, ?, ?, ?, ?)");
ps.setString(1, textField1.getText());
ps.setString(2, textField2.getText());
ps.setString(3, textField3.getText());
ps.setString(4, textField4.getText());
ps.setString(5, textField5.getText());
ps.executeUpdate();
JOptionPane.showMessageDialog(this, "添加成功!");
textField1.setText("");
textField2.setText("");
textField3.setText("");
textField4.setText("");
textField5.setText("");
} catch (Exception ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(this, "添加失败!");
}
}
// 处理删除按钮事件
else if (e.getSource() == button2) {
try {
PreparedStatement ps = conn.prepareStatement("DELETE FROM books WHERE book_id = ?");
ps.setString(1, textField1.getText());
int result = ps.executeUpdate();
if (result > 0) {
JOptionPane.showMessageDialog(this, "删除成功!");
textField1.setText("");
textField2.setText("");
textField3.setText("");
textField4.setText("");
textField5.setText("");
} else {
JOptionPane.showMessageDialog(this, "删除失败!");
}
} catch (Exception ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(this, "删除失败!");
}
}
// 处理更新按钮事件
else if (e.getSource() == button3) {
try {
PreparedStatement ps = conn.prepareStatement("UPDATE books SET book_name = ?, author = ?, publisher = ?, reader_id = ? WHERE book_id = ?");
ps.setString(1, textField2.getText());
ps.setString(2, textField3.getText());
ps.setString(3, textField4.getText());
ps.setString(4, textField5.getText());
ps.setString(5, textField1.getText());
int result = ps.executeUpdate();
if (result > 0) {
JOptionPane.showMessageDialog(this, "更新成功!");
textField1.setText("");
textField2.setText("");
textField3.setText("");
textField4.setText("");
textField5.setText("");
} else {
JOptionPane.showMessageDialog(this, "更新失败!");
}
} catch (Exception ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(this, "更新失败!");
}
}
// 处理查询按钮事件
else if (e.getSource() == button4) {
try {
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM books");
table.setModel(new ResultSetTableModel(rs));
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
public static void main(String[] args) {
new LibrarySystem();
}
}
class ResultSetTableModel extends AbstractTableModel {
private ResultSet rs;
private ResultSetMetaData rsmd;
public ResultSetTableModel(ResultSet rs) throws SQLException {
this.rs = rs;
rsmd = rs.getMetaData();
}
@Override
public int getRowCount() {
try {
rs.last();
return rs.getRow();
} catch (Exception ex) {
ex.printStackTrace();
return 0;
}
}
@Override
public int getColumnCount() {
try {
return rsmd.getColumnCount();
} catch (Exception ex) {
ex.printStackTrace();
return 0;
}
}
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
try {
rs.absolute(rowIndex + 1);
return rs.getObject(columnIndex + 1);
} catch (Exception ex) {
ex.printStackTrace();
return null;
}
}
@Override
public String getColumnName(int columnIndex) {
try {
return rsmd.getColumnLabel(columnIndex + 1);
} catch (Exception ex) {
ex.printStackTrace();
return null;
}
}
}
```
上述代码中,我们使用了Swing库中的JFrame、JPanel、JLabel、JTextField、JButton、JTable和JScrollPane组件来实现用户界面。其中,JTable组件用于显示图书和读者的信息,JScrollPane组件用于添加滚动条。我们还使用了JOptionPane组件来显示提示信息。
我们使用了MySQL数据库来存储图书和读者的信息。在代码中,我们创建了一个名为“library”的数据库,其中包含一个名为“books”的表,该表包含五个字段:图书编号、图书名称、作者、出版社和读者编号。我们在代码中使用了JDBC API来连接数据库、执行SQL语句和处理结果集。注意,在实际使用中,需要根据自己的需求修改数据库连接信息和SQL语句。
我们还创建了一个名为“ResultSetTableModel”的类,该类继承了AbstractTableModel类,并实现了TableModel接口。该类用于将ResultSet对象转换为TableModel对象,以便在JTable中显示查询结果。
你可以根据自己的需求修改代码,并根据需要添加更多的功能,例如借书、还书、查询读者信息等等。
相关推荐
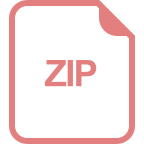
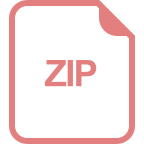














