pyqt5 graphicsView 加载显示图片,在图片上鼠标框选红色矩形并返回矩形在图片上的坐标
时间: 2023-12-22 08:05:44 浏览: 30
可以通过在 `GraphicsView` 上重载 `mousePressEvent` 和 `mouseReleaseEvent` 方法实现鼠标框选矩形的功能,然后通过计算矩形在图片上的坐标来返回。
下面是一个示例代码:
```python
import sys
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.QtCore import *
class GraphicsView(QGraphicsView):
def __init__(self):
super().__init__()
self.setScene(QGraphicsScene(self))
self.setDragMode(QGraphicsView.ScrollHandDrag)
self.rubber_band = QRubberBand(QRubberBand.Rectangle, self)
self.origin = QPoint()
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self.origin = event.pos()
self.rubber_band.setGeometry(QRect(self.origin, QSize()))
self.rubber_band.show()
super().mousePressEvent(event)
def mouseMoveEvent(self, event):
if self.rubber_band.isVisible():
self.rubber_band.setGeometry(QRect(self.origin, event.pos()).normalized())
super().mouseMoveEvent(event)
def mouseReleaseEvent(self, event):
if event.button() == Qt.LeftButton:
self.rubber_band.hide()
rect = self.mapToScene(self.rubber_band.geometry()).boundingRect()
rect = QRectF(rect.x(), rect.y(), rect.width(), rect.height())
print("Selected rect: ", rect)
super().mouseReleaseEvent(event)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setGeometry(100, 100, 800, 600)
self.view = GraphicsView()
self.setCentralWidget(self.view)
self.load_image("test.png")
def load_image(self, file_path):
pixmap = QPixmap(file_path)
self.view.scene().addPixmap(pixmap)
self.view.fitInView(pixmap.rect(), Qt.KeepAspectRatio)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上面的代码中,我们重载了 `GraphicsView` 的 `mousePressEvent`、`mouseMoveEvent` 和 `mouseReleaseEvent` 方法,当按下鼠标左键时就开始绘制红色矩形,当松开鼠标左键时就计算矩形在图片上的坐标并输出。 `QRubberBand` 是一个用于绘制矩形的部件,我们在 `GraphicsView` 上创建一个 `QRubberBand` 对象,并在 `mousePressEvent` 事件中设置其起始位置和大小为零,然后在 `mouseMoveEvent` 中更新其大小以实现绘制矩形的效果。最后,在 `mouseReleaseEvent` 事件中隐藏矩形,并将其坐标映射到场景坐标系中,然后使用 `boundingRect` 方法获取矩形的位置和大小信息,将其转换为 `QRectF` 对象并输出即可。
相关推荐
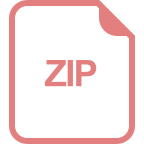
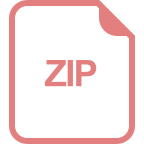








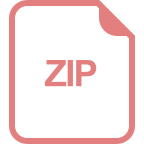
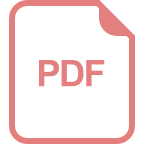
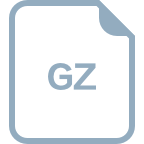
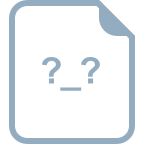