python用函数输入一批学生成绩 求平均成绩和最高分
时间: 2024-02-28 08:24:02 浏览: 163
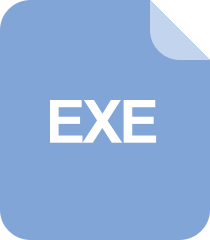
输入一批学生成绩,求出最高分.exe
可以这样实现:
```python
def calculate_scores(scores):
total_scores = sum(scores)
average_score = total_scores / len(scores)
max_score = max(scores)
return average_score, max_score
# 调用函数
scores = [80, 90, 85, 70, 95]
average_score, max_score = calculate_scores(scores)
print("平均成绩:", average_score)
print("最高分:", max_score)
```
代码中,首先定义了一个 `calculate_scores` 函数,该函数接收一个列表参数 `scores`,并返回平均成绩和最高分。在函数中,使用 `sum` 函数求出所有成绩的总分,然后除以成绩的数量得到平均成绩。使用 `max` 函数求出最高分。最后,将平均成绩和最高分作为元组返回。
调用函数时,先定义一个学生成绩的列表 `scores`,然后将其作为参数传递给 `calculate_scores` 函数。最后,使用两个变量 `average_score` 和 `max_score` 分别接收函数返回的平均成绩和最高分,并打印输出。
阅读全文
相关推荐



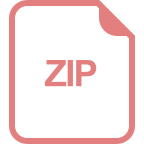

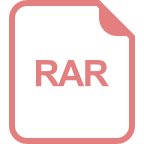
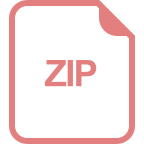
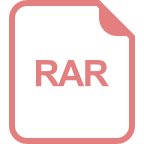
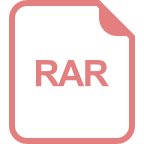
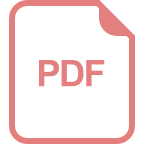
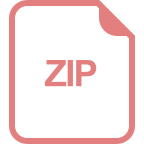
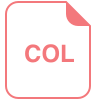
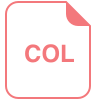
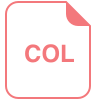
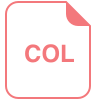
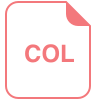