vue点击子元素不触发父元素
时间: 2023-08-12 13:06:38 浏览: 149
可以在子元素的点击事件中加上 `event.stopPropagation()` 方法,以阻止事件冒泡到父元素,例如:
```html
<template>
<div class="parent" @click="parentClick">
<div class="child" @click="childClick"></div>
</div>
</template>
<script>
export default {
methods: {
parentClick() {
console.log('parent clicked');
},
childClick(event) {
event.stopPropagation();
console.log('child clicked');
},
},
};
</script>
```
这样,当点击子元素时,只会触发子元素的点击事件,父元素的点击事件不会被触发。
相关问题
vue2 子元素传递给父元素内容
在Vue2中,可以使用自定义事件来实现子组件向父组件传递数据。具体步骤如下:
1. 在子组件中,使用 `$emit` 方法触发自定义事件,并传递数据。例如:
```
<template>
<button @click="$emit('send', 'hello')">发送</button>
</template>
```
上述代码定义了一个名为 `send` 的自定义事件,并传递了字符串 `'hello'`。
2. 在父组件中,使用 `v-on` 指令监听子组件触发的自定义事件,并在对应的方法中接收数据。例如:
```
<template>
<child-component @send="handleSend"></child-component>
<div>{{ message }}</div>
</template>
<script>
export default {
data() {
return {
message: ''
}
},
methods: {
handleSend(data) {
this.message = data
}
}
}
</script>
```
上述代码中,`<child-component>` 组件触发了 `send` 事件,父组件中的 `handleSend` 方法接收到了传递的数据,并将数据赋值给了 `message` 属性。最后,父组件中的 `<div>` 标签中显示了 `message` 的值。
通过以上步骤,就可以在Vue2中实现子组件向父组件传递数据了。
vue2 子元素传递给父元素内容 怎么传递
在Vue2中,可以使用自定义事件来实现子组件向父组件传递数据。具体步骤如下:
1. 在子组件中,使用 `$emit` 方法触发自定义事件,并传递数据。例如:
```
<template>
<button @click="$emit('send', 'hello')">发送</button>
</template>
```
上述代码定义了一个名为 `send` 的自定义事件,并传递了字符串 `'hello'`。
2. 在父组件中,使用 `v-on` 指令监听子组件触发的自定义事件,并在对应的方法中接收数据。例如:
```
<template>
<child-component @send="handleSend"></child-component>
<div>{{ message }}</div>
</template>
<script>
export default {
data() {
return {
message: ''
}
},
methods: {
handleSend(data) {
this.message = data
}
}
}
</script>
```
上述代码中,`<child-component>` 组件触发了 `send` 事件,父组件中的 `handleSend` 方法接收到了传递的数据,并将数据赋值给了 `message` 属性。最后,父组件中的 `<div>` 标签中显示了 `message` 的值。
通过以上步骤,就可以在Vue2中实现子组件向父组件传递数据了。
阅读全文
相关推荐






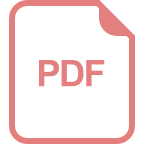
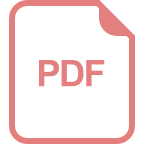





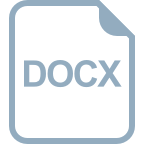