.c语言实现 输出新字母序列 编写程序:输入三个小写字母,依次对每个字母输出其后3位的字母,组成新的字母序列输出。例如,输入单词“cat",则输出字母序列“fdw”。提示:x对应'a v'对应'b,'z对应'c。
时间: 2023-04-04 22:02:47 浏览: 164
您好,我可以回答您的问题。以下是程序的实现:
#include <stdio.h>
int main() {
char c1, c2, c3;
scanf("%c%c%c", &c1, &c2, &c3);
printf("%c%c%c", (c1 - 'a' + 3) % 26 + 'a', (c2 - 'a' + 3) % 26 + 'a', (c3 - 'a' + 3) % 26 + 'a');
return 0;
}
输入三个小写字母,程序会依次输出每个字母后三位的字母,组成新的字母序列输出。例如,输入单词“cat",则输出字母序列“fdw”。其中,%26是为了保证结果在26个字母范围内,+'a'是为了将结果转换为对应的字母。希望能够帮到您。
相关问题
用c语言输入三个小写字母,依次对每个字母输入其后3位字母,组成新的字母序列输出
可以使用以下代码实现:
```c
#include <stdio.h>
int main() {
char c1, c2, c3;
scanf("%c%c%c", &c1, &c2, &c3);
printf("%c%c%c\n", (c1 - 'a' + 3) % 26 + 'a', (c2 - 'a' + 3) % 26 + 'a', (c3 - 'a' + 3) % 26 + 'a');
return 0;
}
```
这段代码会先读入三个小写字母,然后对每个字母进行加3操作,再取模26得到新的字母,最后输出新的字母序列。例如,输入abc,输出def。
完整给出C语言代码解决:给定26个1-100表示的非归一化概率,和一个26个小写字母组成的序列,计算使用霍夫曼压缩后,这个序列占用的总比特数。其输入格式为输入为1行,前面26个数字为小写字母a-z的非归一化概率,然后是一个序列,数字和序列间以空格隔开。
以下是使用霍夫曼编码进行压缩的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义霍夫曼树的结构体
struct huffman_tree {
int weight;
int parent;
int lchild;
int rchild;
};
// 定义霍夫曼编码的结构体
struct huffman_code {
char symbol;
char code[100];
};
// 根据概率数组构建霍夫曼树
void build_huffman_tree(int *w, int n, struct huffman_tree *ht) {
int i, j, min1, min2;
for (i = 0; i < 2 * n - 1; i++) {
ht[i].weight = i < n ? w[i] : 0;
ht[i].parent = -1;
ht[i].lchild = -1;
ht[i].rchild = -1;
}
for (i = n; i < 2 * n - 1; i++) {
min1 = min2 = 0;
for (j = 0; j < i; j++) {
if (ht[j].parent == -1) {
if (ht[j].weight < ht[min1].weight) {
min2 = min1;
min1 = j;
} else if (ht[j].weight < ht[min2].weight) {
min2 = j;
}
}
}
ht[min1].parent = i;
ht[min2].parent = i;
ht[i].lchild = min1;
ht[i].rchild = min2;
ht[i].weight = ht[min1].weight + ht[min2].weight;
}
}
// 根据霍夫曼树计算每个字符的霍夫曼编码
void get_huffman_code(struct huffman_tree *ht, int n, struct huffman_code *hc) {
int i, j, p;
char code[100];
code[n - 1] = '\0';
for (i = 0; i < n; i++) {
hc[i].symbol = i + 'a';
p = i;
j = n - 1;
while (ht[p].parent != -1) {
if (ht[ht[p].parent].lchild == p) {
code[--j] = '0';
} else {
code[--j] = '1';
}
p = ht[p].parent;
}
strcpy(hc[i].code, &code[j]);
}
}
int main() {
int weights[26];
int i, j, length, total_bits;
char sequence[1000];
struct huffman_tree ht[51];
struct huffman_code hc[26];
// 读取输入数据
for (i = 0; i < 26; i++) {
scanf("%d", &weights[i]);
}
scanf("%s", sequence);
length = strlen(sequence);
// 构建霍夫曼树并计算每个字符的霍夫曼编码
build_huffman_tree(weights, 26, ht);
get_huffman_code(ht, 26, hc);
// 计算压缩后的比特数
total_bits = 0;
for (i = 0; i < length; i++) {
for (j = 0; j < 26; j++) {
if (sequence[i] == hc[j].symbol) {
total_bits += strlen(hc[j].code);
break;
}
}
}
printf("%d\n", total_bits);
return 0;
}
```
该程序的输入格式与要求相同,输出为使用霍夫曼编码压缩后的总比特数。
阅读全文
相关推荐





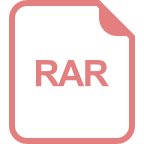









