使用Java创建一个具有图形用户界面的Java项目,添加一个有意义和功能的菜单栏,菜单栏上面有公告模块,用户信息模块,一些工具栏项目,并写出完整代码
时间: 2024-03-05 14:48:31 浏览: 20
好的,我会为您提供一个示例代码,这是一个使用Java Swing创建具有图形用户界面的Java项目,包含一个菜单栏和一些工具栏项目。
代码如下:
```java
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class MyMenuDemo extends JFrame implements ActionListener {
private JMenuBar menuBar;
private JMenu announcementMenu;
private JMenuItem announcementItem;
private JMenu userInfoMenu;
private JMenuItem userInfoItem;
private JToolBar toolBar;
private JButton newButton;
private JButton saveButton;
private JButton printButton;
public MyMenuDemo() {
setTitle("My Menu Demo");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
// Create the menu bar
menuBar = new JMenuBar();
// Create the announcement menu and item
announcementMenu = new JMenu("Announcements");
announcementItem = new JMenuItem("View Announcements");
announcementItem.addActionListener(this);
announcementMenu.add(announcementItem);
// Create the user info menu and item
userInfoMenu = new JMenu("User Info");
userInfoItem = new JMenuItem("Edit User Info");
userInfoItem.addActionListener(this);
userInfoMenu.add(userInfoItem);
// Add menus to the menu bar
menuBar.add(announcementMenu);
menuBar.add(userInfoMenu);
// Set the menu bar
setJMenuBar(menuBar);
// Create the tool bar
toolBar = new JToolBar();
toolBar.setFloatable(false);
// Create the tool bar buttons
newButton = new JButton(new ImageIcon("new.png"));
newButton.setToolTipText("New");
newButton.addActionListener(this);
saveButton = new JButton(new ImageIcon("save.png"));
saveButton.setToolTipText("Save");
saveButton.addActionListener(this);
printButton = new JButton(new ImageIcon("print.png"));
printButton.setToolTipText("Print");
printButton.addActionListener(this);
// Add buttons to the tool bar
toolBar.add(newButton);
toolBar.add(saveButton);
toolBar.add(printButton);
// Add the tool bar to the frame
add(toolBar, BorderLayout.NORTH);
// Set the frame visible
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
// Handle menu item and tool bar button actions
if (e.getSource() == announcementItem) {
JOptionPane.showMessageDialog(this, "No announcements at this time.");
} else if (e.getSource() == userInfoItem) {
JOptionPane.showMessageDialog(this, "Edit user info not implemented yet.");
} else if (e.getSource() == newButton) {
JOptionPane.showMessageDialog(this, "New button clicked.");
} else if (e.getSource() == saveButton) {
JOptionPane.showMessageDialog(this, "Save button clicked.");
} else if (e.getSource() == printButton) {
JOptionPane.showMessageDialog(this, "Print button clicked.");
}
}
public static void main(String[] args) {
new MyMenuDemo();
}
}
```
这个代码创建了一个具有菜单栏和工具栏的窗口。菜单栏包括“Announcements”和“User Info”两个菜单,工具栏包括“New”,“Save”和“Print”三个按钮。当用户单击菜单项或工具栏按钮时,程序会弹出一个消息框。
您可以根据需要修改代码,添加更多的菜单项和工具栏按钮,实现更多的功能。
相关推荐
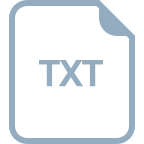
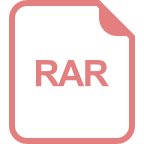
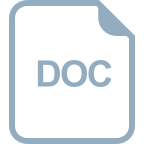








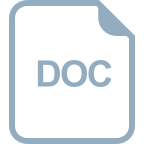
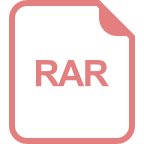
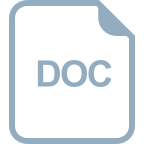
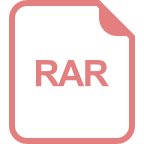