编写程序,输入月薪数a,计算并输出税率、应缴税款和实得奖金数。工薪所得扣除标准提高到3500元。
时间: 2024-12-17 13:38:14 浏览: 28
编写这样一个程序的基本思路如下:
首先,需要定义几个变量:
- `a`: 输入的月薪数
- `threshold`: 扣除标准,这里是3500元
- `tax_rates`: 税率列表(通常按阶梯累进税率划分)
- `tax_brackets`: 级别区间范围
- `tax_amount`: 应缴税款
- `deduction`: 费用扣除
- `after_tax`: 实得奖金数
算法步骤如下:
1. 检查`a`是否超过`threshold`,如果不超过,则直接设置`deduction`为0,因为不需要缴税。
2. 如果超过,从`a`中减去`threshold`得到`taxable_income`(应税所得)。
3. 遍历税率表,找出适用的税率级别,计算对应级别的税费。
4. 根据税率级别计算税额,更新`tax_amount`。
5. 更新`deduction`,包括基本扣除和其他可能的专项扣除。
6. 最后,计算`after_tax`,等于`a`减去`tax_amount`和`deduction`。
以下是简单的Python示例代码(假设税率为百分比形式,例如20%):
```python
def calculate_tax(a):
threshold = 3500
tax_rates = [0, 3%, 10%, 20%] # 假设简化税率
tax_brackets = [(0, threshold), (threshold, a * 2), (a * 2, float('inf'))]
taxable_income = max(0, a - threshold)
for rate_range in tax_brackets:
if taxable_income > rate_range[1]:
tax_rate = rate_range[0]
break
else:
tax_rate = rate_range[0]
taxable_income -= rate_range[1]
tax_amount = taxable_income * tax_rate
deduction = threshold + ... # 其他扣除项,这里省略
after_tax = a - tax_amount - deduction
return tax_rate, tax_amount, after_tax
# 示例
a = float(input("请输入您的月薪:"))
rate, amount, real_wage = calculate_tax(a)
print(f"税率: {rate*100}%\n应缴税款: {amount}\n实得奖金数: {real_wage}")
```
阅读全文
相关推荐



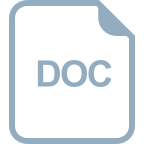










