用c语言写静态链表代码
时间: 2023-04-09 16:02:32 浏览: 69
我可以回答这个问题。以下是一个用C语言写的静态链表代码的示例:
#include <stdio.h>
#define MAXSIZE 100
typedef struct {
int data;
int next;
} Node;
Node List[MAXSIZE];
int main() {
int head, n, i, j, k;
printf("请输入链表长度:");
scanf("%d", &n);
printf("请输入链表元素:\n");
for (i = 0; i < n; i++) {
scanf("%d", &List[i].data);
List[i].next = i + 1;
}
List[n - 1].next = -1;
head = 0;
printf("链表的内容为:\n");
i = head;
while (i != -1) {
printf("%d ", List[i].data);
i = List[i].next;
}
printf("\n");
return 0;
}
相关问题
用C语言生成静态链表并加上注释
下面是一个用C语言生成静态链表的示例代码,每行代码都添加了注释以便理解:
```c
#include <stdio.h>
// 定义链表结构体
struct Node {
int data; // 数据域
int next; // 指针域,表示下一个节点的位置
};
int main() {
// 定义静态链表
struct Node list[5] = {
{1, 1}, // 第一个节点的数据为1,下一个节点的位置为1(因为是第二个节点,所以指向自己)
{2, 3}, // 第二个节点的数据为2,下一个节点的位置为3
{3, 4}, // 第三个节点的数据为3,下一个节点的位置为4
{4, 2}, // 第四个节点的数据为4,下一个节点的位置为2
{5, -1} // 第五个节点的数据为5,下一个节点的位置为-1(表示链表结束)
};
// 输出链表中的元素
int i = 0;
while (list[i].next != -1) { // 如果下一个节点的位置不是-1(即还有下一个节点)
printf("%d ", list[i].data); // 输出当前节点的数据
i = list[i].next; // 将当前节点的下一个节点作为下一次循环的当前节点
}
printf("%d\n", list[i].data); // 输出最后一个节点的数据
return 0;
}
```
这个示例代码定义了一个长度为5的静态链表,其中每个节点都包含一个数据域和一个指针域。在生成链表时,每个节点的指针域都指向下一个节点的位置,最后一个节点的指针域为-1,表示链表的结束。在遍历链表时,代码通过不断修改当前节点的指针域来遍历整个链表,并输出每个节点的数据域。
用c语言写一个静态链表
可以使用结构体和指针来实现静态链表。首先定义一个结构体,包含数据和指向下一个节点的指针。然后定义一个数组来存储这些节点,每个节点的下标就是它的地址。最后,使用指针来操作这些节点,实现链表的功能。
以下是一个简单的静态链表的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct Node {
int data;
int next;
} Node;
Node list[MAX_SIZE];
int head = -1;
void init() {
for (int i = ; i < MAX_SIZE; i++) {
list[i].next = -2;
}
}
void add(int data) {
int i = ;
while (list[i].next != -2) {
i = list[i].next;
}
list[i].next = head;
head = i + 1;
list[head - 1].data = data;
}
void print() {
int i = head;
while (i != -1) {
printf("%d ", list[i - 1].data);
i = list[i - 1].next;
}
printf("\n");
}
int main() {
init();
add(1);
add(2);
add(3);
print();
return ;
}
```
这个代码实现了一个简单的静态链表,可以添加数据并打印链表中的所有数据。
相关推荐
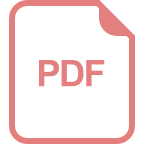
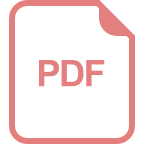
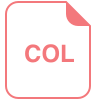
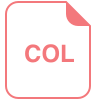
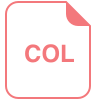
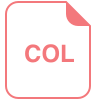
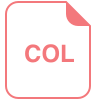







