java集成gpt
时间: 2023-07-03 13:28:32 浏览: 76
Java本身不支持GPT模型,但可以通过使用Java调用Python GPT库,实现GPT模型的集成。下面是一个简单的示例代码:
1. 首先,你需要安装Python和相应的GPT库,例如transformers库。
2. 编写一个Python脚本,实现GPT模型的加载和对话功能,例如以下代码:
```python
from transformers import AutoModelForCausalLM, AutoTokenizer
import torch
tokenizer = AutoTokenizer.from_pretrained("microsoft/DialoGPT-medium")
model = AutoModelForCausalLM.from_pretrained("microsoft/DialoGPT-medium")
def generate_response(input_text):
input_ids = tokenizer.encode(input_text + tokenizer.eos_token, return_tensors='pt')
chat_history_ids = model.generate(input_ids, max_length=1000, pad_token_id=tokenizer.eos_token_id)
response = tokenizer.decode(chat_history_ids[:, input_ids.shape[-1]:][0], skip_special_tokens=True)
return response
```
3. 在Java中调用Python脚本,实现与GPT模型的交互。可以使用Python的subprocess模块来实现。例如以下代码:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class GPTChatbot {
public static void main(String[] args) throws IOException {
// Initialize Python process
Process process = Runtime.getRuntime().exec("python gpt.py");
// Get input and output streams
BufferedReader input = new BufferedReader(new InputStreamReader(process.getInputStream()));
BufferedReader error = new BufferedReader(new InputStreamReader(process.getErrorStream()));
// Send input to Python process
String userInput = "Hello";
process.getOutputStream().write(userInput.getBytes());
process.getOutputStream().flush();
// Receive output from Python process
String output = input.readLine();
System.out.println(output);
// Close input and error streams
input.close();
error.close();
// Wait for Python process to complete
try {
process.waitFor();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
这个例子中,Java调用了一个名为“gpt.py”的Python脚本,然后向Python脚本发送一个字符串“Hello”,并从Python脚本获取回应。你可以根据需要修改这个代码,实现更复杂的对话逻辑。
相关推荐
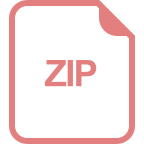
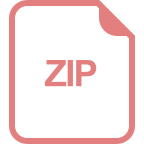














