java rpc示例
时间: 2023-07-07 15:05:49 浏览: 113
Java RPC(Remote Procedure Call)是一种远程过程调用技术,它允许我们在不同的进程或者不同的机器之间进行通信。Java中有多种实现RPC的方式,其中比较流行的有RMI和gRPC。
下面是一个简单的Java RMI示例:
首先,我们需要定义一个远程接口,这个接口中定义了我们需要远程调用的方法:
```java
import java.rmi.Remote;
import java.rmi.RemoteException;
public interface MyRemoteInterface extends Remote {
public String sayHello() throws RemoteException;
}
```
然后,我们需要创建一个远程对象,这个对象实现了我们定义的接口:
```java
import java.rmi.RemoteException;
import java.rmi.server.UnicastRemoteObject;
public class MyRemoteObject extends UnicastRemoteObject implements MyRemoteInterface {
public MyRemoteObject() throws RemoteException {}
public String sayHello() throws RemoteException {
return "Hello, world!";
}
}
```
接下来,我们需要创建一个RMI注册表,将我们的远程对象注册到这个注册表中:
```java
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
public class MyRemoteServer {
public static void main(String[] args) {
try {
MyRemoteObject obj = new MyRemoteObject();
Registry registry = LocateRegistry.createRegistry(1099);
registry.bind("MyRemoteObject", obj);
System.out.println("Remote object bound to registry.");
} catch (Exception e) {
System.err.println("Error: " + e.getMessage());
e.printStackTrace();
}
}
}
```
最后,我们需要编写一个客户端程序,从RMI注册表中查找我们的远程对象,并调用其中的方法:
```java
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
public class MyRemoteClient {
public static void main(String[] args) {
try {
Registry registry = LocateRegistry.getRegistry("localhost");
MyRemoteInterface obj = (MyRemoteInterface) registry.lookup("MyRemoteObject");
String result = obj.sayHello();
System.out.println(result);
} catch (Exception e) {
System.err.println("Error: " + e.getMessage());
e.printStackTrace();
}
}
}
```
运行这个程序,我们应该可以看到输出结果为:
```
Remote object bound to registry.
Hello, world!
```
这就是一个基本的Java RMI示例。通过RMI,我们可以在不同的Java虚拟机中进行远程方法调用,从而实现分布式计算。
阅读全文
相关推荐


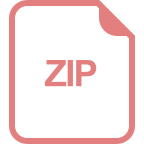
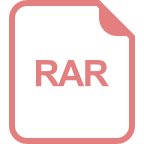
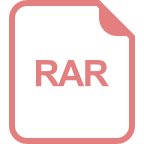
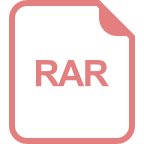
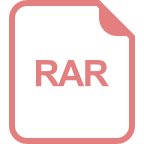
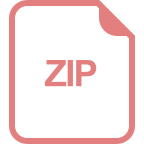
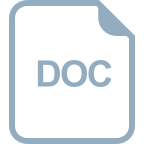
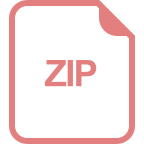
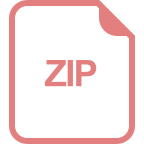
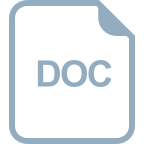
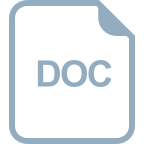
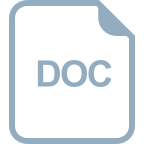
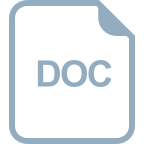
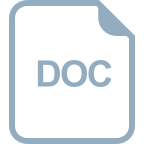