定义数组存放10个学生的成绩(成绩值自己设定),将成绩从大到小排序,计算并显示输出成绩总和,平均成绩,最小成绩,最大成绩
时间: 2024-10-13 20:07:51 浏览: 37
首先,我们需要创建一个包含10个学生成绩的数组,并初始化成绩。然后我们可以使用`Arrays.sort()`函数对数组进行降序排列。接下来,我们将分别计算总和、平均值、最小值和最大值。以下是用Java实现的一个示例:
```java
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
// 初始化成绩数组,成绩值自己设定
int[] scores = {85, 92, 78, 96, 75, 98, 88, 90, 80, 83};
// 对成绩数组进行降序排序
Arrays.sort(scores);
int[] sortedScores = new int[scores.length];
System.arraycopy(scores, 0, sortedScores, sortedScores.length - scores.length, scores.length); // 将原数组反转得到降序数组
// 计算成绩相关统计信息
double totalScore = 0;
for (int score : sortedScores) {
totalScore += score;
}
double averageScore = totalScore / sortedScores.length;
int minScore = sortedScores[0];
int maxScore = sortedScores[sortedScores.length - 1];
// 输出结果
System.out.println("成绩排序后的列表:");
for (int i : sortedScores) {
System.out.print(i + " ");
}
System.out.println("\n总成绩: " + totalScore);
System.out.println("平均成绩: " + averageScore);
System.out.println("最小成绩: " + minScore);
System.out.println("最大成绩: " + maxScore);
}
}
```
运行此程序后,它将按照成绩从高到低展示,并输出相应的总和、平均值、最小值和最大值。
阅读全文
相关推荐
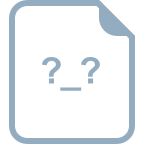
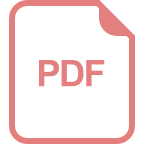
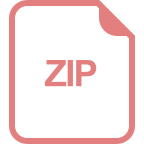








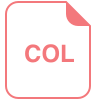
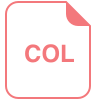
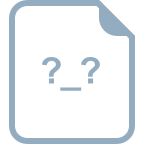
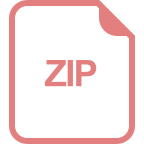
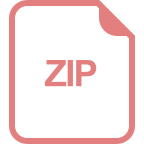
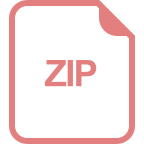
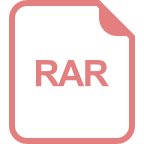