java jSerialComm 多线程
时间: 2023-03-04 16:23:02 浏览: 96
jSerialComm 是一个Java串口通信库,可以通过该库来实现串口通信的功能。如果需要在多线程环境下使用jSerialComm,可以考虑使用Java的多线程机制。
以下是使用jSerialComm实现多线程串口通信的示例代码:
```java
import com.fazecast.jSerialComm.SerialPort;
public class SerialCommThread extends Thread {
private SerialPort serialPort;
private boolean running;
public SerialCommThread(SerialPort serialPort) {
this.serialPort = serialPort;
this.running = false;
}
public void run() {
running = true;
while (running) {
// 读取串口数据
byte[] buffer = new byte[1024];
int numBytes = serialPort.readBytes(buffer, buffer.length);
// 处理数据
if (numBytes > 0) {
String message = new String(buffer, 0, numBytes);
System.out.println(message);
}
}
}
public void stopThread() {
running = false;
}
}
public class Main {
public static void main(String[] args) {
SerialPort serialPort = SerialPort.getCommPort("/dev/ttyACM0");
serialPort.openPort();
serialPort.setBaudRate(9600);
SerialCommThread thread = new SerialCommThread(serialPort);
thread.start();
// 在主线程中发送串口数据
byte[] buffer = "Hello, world!".getBytes();
serialPort.writeBytes(buffer, buffer.length);
// 在主线程中等待一段时间,然后停止串口线程
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
thread.stopThread();
serialPort.closePort();
}
}
```
在这个例子中,SerialCommThread类继承了Thread类,实现了一个串口通信的线程。在run方法中,使用serialPort.readBytes方法读取串口数据,并进行处理。stopThread方法用于停止线程。
在主线程中,创建一个SerialCommThread对象,并启动线程。然后使用serialPort.writeBytes方法向串口发送数据。在一定时间后,调用thread.stopThread方法停止串口线程,并使用serialPort.closePort方法关闭串口。
相关推荐
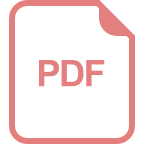
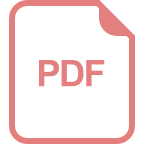
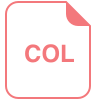
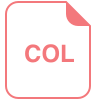
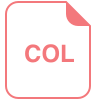
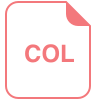
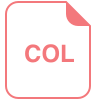






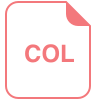