java输出五子棋程序
时间: 2023-06-05 17:07:24 浏览: 68
好的,我可以为您分享一个基于Java编写的五子棋程序。以下为代码:
```
import java.util.Scanner;
public class GobangGame {
private static char[][] board;
private static final int ROW = 15;
private static final int COL = 15;
private static char playerOne = 'X';
private static char playerTwo = 'O';
public static void main(String[] args) {
initBoard();
printBoard();
Scanner scanner = new Scanner(System.in);
int x, y;
char currentPlayer = playerOne;
while (true) {
System.out.printf("Player %s, please enter the coordinate row and column you want to place the chess piece(separated by space):", currentPlayer);
x = scanner.nextInt();
y = scanner.nextInt();
if (isValid(x, y)) {
board[x][y] = currentPlayer;
printBoard();
if (isWin(x, y)) {
System.out.printf("Congratulations! Player %s won the game!", currentPlayer);
break;
}
if (isFull()) {
System.out.println("The game ended in a draw!");
break;
}
currentPlayer = currentPlayer == playerOne ? playerTwo : playerOne;
} else {
System.out.println("Illegal move, please try again.");
}
}
}
public static void initBoard() {
board = new char[ROW][COL];
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
board[i][j] = '+';
}
}
}
public static void printBoard() {
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
public static boolean isValid(int x, int y) {
if (x < 0 || x >= ROW || y < 0 || y >= COL) {
return false;
}
if (board[x][y] != '+') {
return false;
}
return true;
}
public static boolean isWin(int x, int y) {
char current = board[x][y];
int count = 1; // count for horizontal
int i, j;
// check horizontal
i = x;
j = y - 1;
while (j >= 0 && board[i][j] == current) {
count++;
j--;
}
j = y + 1;
while (j < COL && board[i][j] == current) {
count++;
j++;
}
if (count >= 5) {
return true;
}
// check vertical
count = 1;
i = x - 1;
j = y;
while (i >= 0 && board[i][j] == current) {
count++;
i--;
}
i = x + 1;
while (i < ROW && board[i][j] == current) {
count++;
i++;
}
if (count >= 5) {
return true;
}
// check diagonal
count = 1;
i = x - 1;
j = y - 1;
while (i >= 0 && j >= 0 && board[i][j] == current) {
count++;
i--;
j--;
}
i = x + 1;
j = y + 1;
while (i < ROW && j < COL && board[i][j] == current) {
count++;
i++;
j++;
}
if (count >= 5) {
return true;
}
// check anti-diagonal
count = 1;
i = x - 1;
j = y + 1;
while (i >= 0 && j < COL && board[i][j] == current) {
count++;
i--;
j++;
}
i = x + 1;
j = y - 1;
while (i < ROW && j >= 0 && board[i][j] == current) {
count++;
i++;
j--;
}
if (count >= 5) {
return true;
}
return false;
}
public static boolean isFull() {
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
if (board[i][j] == '+') {
return false;
}
}
}
return true;
}
}
```
希望这个程序能够帮助您学习五子棋的编写。如果还有其他问题,可以随时向我提出。
相关推荐
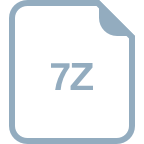
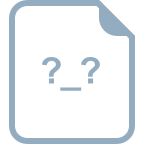
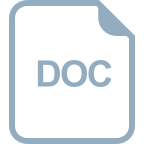















