如何获取当前城市信息和天气并产生与天气对应的图片
时间: 2024-06-08 08:07:46 浏览: 116
获取当前城市信息和天气需要使用天气API。常用的天气API有心知天气、和风天气等,这里以心知天气为例进行介绍。
首先,需要在心知天气官网注册账号并创建应用,获取应用的API Key。然后,可以使用API进行城市信息和天气的查询。例如,以下是使用心知天气API查询北京市的天气信息的示例:
```
import requests
api_key = "your_api_key"
city = "beijing"
url = f"https://api.seniverse.com/v3/weather/now.json?key={api_key}&location={city}&language=zh-Hans&unit=c"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
weather = data["results"][0]["now"]["text"]
temperature = data["results"][0]["now"]["temperature"]
print(f"{city}当前天气为{weather},温度为{temperature}℃。")
else:
print("查询失败。")
```
上述代码中,`api_key`是在心知天气官网创建应用时获取的API Key,`city`是需要查询的城市名称。`requests.get(url)`发送GET请求获取天气信息,并解析返回的JSON数据获取当前天气和温度信息。
接下来,可以使用Python的PIL库(Python Imaging Library)生成与天气对应的图片。例如,以下是根据天气状况生成不同图片的示例:
```
from PIL import Image, ImageDraw, ImageFont
# 根据天气状况生成不同图片
def generate_weather_image(weather):
if weather == "晴":
background_color = (255, 255, 204) #黄色
image_text = "☀️"
elif weather == "多云":
background_color = (153, 204, 255) #蓝色
image_text = "🌥️"
elif weather == "阴":
background_color = (192, 192, 192) #灰色
image_text = "☁️"
elif weather == "雨":
background_color = (0, 102, 153) #深蓝色
image_text = "🌧️"
else:
background_color = (255, 255, 255) #白色
image_text = "?"
# 创建画布
image = Image.new("RGB", (200, 200), background_color)
# 在画布上添加文字
draw = ImageDraw.Draw(image)
font = ImageFont.truetype("arial.ttf", 100)
draw.text((50, 50), image_text, (255, 255, 255), font=font)
return image
# 测试生成图片
image = generate_weather_image("晴")
image.show()
```
上述代码中,`generate_weather_image`函数根据不同的天气状况生成不同的图片,例如,如果天气为“晴”,则生成黄色背景,画上“☀️”文字的图片。`PIL.Image.new`创建画布,`PIL.ImageDraw.Draw`在画布上添加文字,`PIL.ImageFont.truetype`指定字体和字号。
最后,可以将获取的天气信息和生成的图片结合起来,例如,以下是获取北京市天气信息并生成对应图片的示例:
```
import requests
from PIL import Image, ImageDraw, ImageFont
api_key = "your_api_key"
city = "beijing"
url = f"https://api.seniverse.com/v3/weather/now.json?key={api_key}&location={city}&language=zh-Hans&unit=c"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
weather = data["results"][0]["now"]["text"]
temperature = data["results"][0]["now"]["temperature"]
print(f"{city}当前天气为{weather},温度为{temperature}℃。")
image = generate_weather_image(weather)
image.show()
else:
print("查询失败。")
```
上述代码中,首先使用心知天气API获取北京市的天气信息,然后根据天气状况生成对应的图片,并使用`image.show()`显示图片。
阅读全文
相关推荐
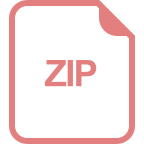
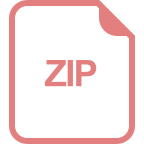
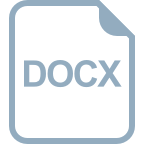
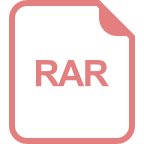
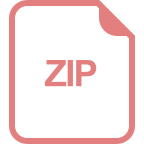
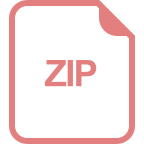
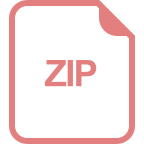
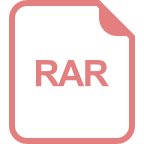
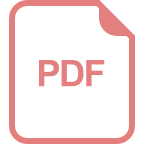
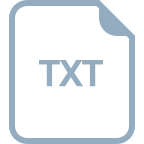
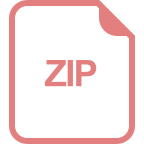
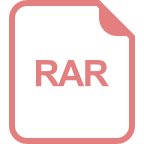
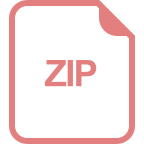
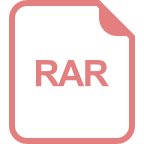
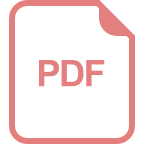
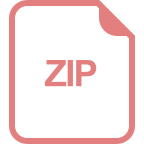