vue3 表格拖拽 js
时间: 2023-06-28 08:12:09 浏览: 48
在Vue3中实现表格拖拽的一个思路是使用HTML5提供的Drag and Drop API,其实现步骤如下:
1. 给表格中的每一列添加draggable属性,并设置对应的列索引。
2. 监听每一列的dragstart事件,并将被拖动的列的信息存储在dataTransfer对象中。
3. 监听表格的dragover事件,并阻止默认行为,同时设置dropEffect为move。
4. 监听表格的drop事件,并获取被拖动列和目标列的索引,然后交换它们的位置。
5. 更新表格数据。
下面是一个简单的实现代码示例:
```html
<template>
<table>
<thead>
<tr>
<th v-for="(col, index) in columns"
:key="index"
:draggable="true"
@dragstart="onDragStart(index)"
@dragover.prevent
@drop="onDrop(index)">
{{ col }}
</th>
</tr>
</thead>
<tbody>
<tr v-for="(row, rowIndex) in rows" :key="rowIndex">
<td v-for="(col, colIndex) in row" :key="colIndex">
{{ col }}
</td>
</tr>
</tbody>
</table>
</template>
<script>
export default {
data() {
return {
columns: ['Name', 'Age', 'Gender'],
rows: [
{ name: 'Alice', age: 25, gender: 'F' },
{ name: 'Bob', age: 30, gender: 'M' },
{ name: 'Charlie', age: 35, gender: 'M' }
]
};
},
methods: {
onDragStart(index) {
// 存储被拖动列的索引
event.dataTransfer.setData('text/plain', index);
},
onDrop(index) {
// 获取被拖动列和目标列的索引
const dragIndex = event.dataTransfer.getData('text/plain');
// 交换列的位置
const temp = this.columns[dragIndex];
this.$set(this.columns, dragIndex, this.columns[index]);
this.$set(this.columns, index, temp);
}
}
};
</script>
```
需要注意的是,这里只是一个简单的示例,实际情况下还需要考虑一些细节,比如拖动时的样式、拖动到非表头位置时的处理等。
相关推荐
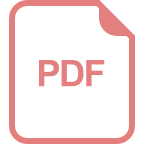
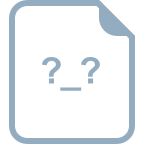














