c++中,求一个矩阵中出现元素最多的个数
时间: 2024-10-22 07:24:31 浏览: 30
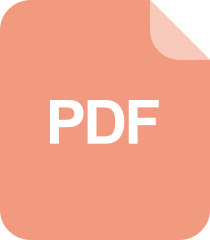
详解C++中的一维数组和二维数组

在C++中,如果你想要计算一个二维矩阵(也称为矩阵)中出现次数最多的元素,你可以采用哈希表(unordered_map或map)和计数器来实现。以下是一个简单的步骤:
1. 定义一个函数接收矩阵作为输入。
2. 遍历矩阵中的每个元素,将元素作为键存储到哈希表中,并更新相应的值(初始为1)。
3. 再次遍历哈希表,找到最大值并记录对应的元素。
4. 返回这个元素的值,即为出现次数最多的元素个数。
```cpp
#include <iostream>
#include <unordered_map>
int countMostFrequent(int matrix[], int rows, int cols) {
std::unordered_map<int, int> frequencyMap;
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
frequencyMap[matrix[i][j]]++;
}
}
int maxCount = 0, mostFrequent = matrix[0][0];
for (const auto& pair : frequencyMap) {
if (pair.second > maxCount) {
maxCount = pair.second;
mostFrequent = pair.first;
}
}
return maxCount;
}
int main() {
int matrix[] = {{1, 1, 2}, {2, 2, 3}, {3, 3, 1}};
int rows = sizeof(matrix) / sizeof(matrix[0]);
int cols = sizeof(matrix[0]) / sizeof(matrix[0][0]);
int mostCommon = countMostFrequent(matrix, rows, cols);
std::cout << "Most frequent element appears " << mostCommon << " times." << std::endl;
return 0;
}
```
在这个例子中,`countMostFrequent` 函数会返回矩阵中元素出现最多次的数量。
阅读全文
相关推荐
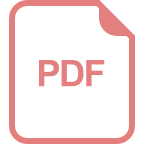
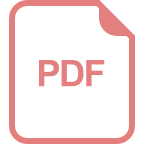
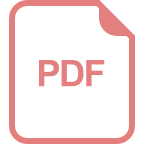
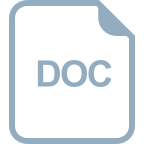
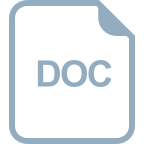
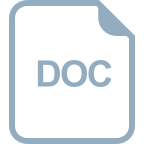
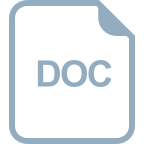










