python提取网页中文字
时间: 2023-10-26 21:06:52 浏览: 67
你可以使用Python的requests和BeautifulSoup库来提取网页中的文字。下面是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 发送请求
url = 'https://www.example.com'
response = requests.get(url)
# 解析HTML
soup = BeautifulSoup(response.content, 'html.parser')
# 提取文字
text = soup.get_text()
# 打印结果
print(text)
```
这个代码会向指定的URL发送一个GET请求,并将返回的HTML内容传递给BeautifulSoup库来解析。然后,使用soup.get_text()方法来提取HTML中的所有文字。最后,将提取的文字打印出来。
相关问题
使用python获取网页中的所有文字
您可以使用Python中的requests和BeautifulSoup库来获取网页中的所有文字。以下是一个示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
text = soup.get_text()
print(text)
```
在这个示例中,我们首先使用requests库获取网页的内容,然后使用BeautifulSoup库将其解析为HTML格式。接着,我们可以使用get_text()方法来提取所有的文本内容,并打印出来。
请注意,这种方法提取的文本内容可能包含一些HTML标签,如<div>和<span>等。如果您需要去除这些标签,请参考BeautifulSoup库的文档,使用相应的方法进行处理。
python爬虫爬取网页文字
在 Python 中,我们可以使用第三方库 requests 和 BeautifulSoup 来爬取网页文字。
首先,我们需要安装这两个库。可以通过 pip 命令来安装。
```
pip install requests
pip install beautifulsoup4
```
然后,我们可以使用 requests 库来获取网页的 HTML 内容,如下所示:
```python
import requests
url = "https://www.example.com"
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print("Failed to retrieve HTML content")
```
这段代码将会获取 https://www.example.com 网页的 HTML 内容,并打印出来。
接下来,我们可以使用 BeautifulSoup 库来解析 HTML 内容,提取出我们需要的文字内容。如下所示:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
text_content = soup.get_text()
print(text_content)
```
这段代码将会使用 BeautifulSoup 解析上一步获取到的 HTML 内容,并提取出网页的文字内容,并打印出来。
相关推荐
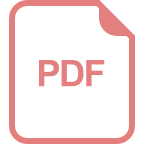
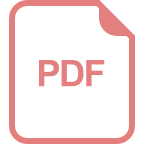
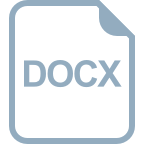












