Winform combobox的下拉部分实现触屏上下滑动
时间: 2023-11-22 21:56:09 浏览: 79
Winform combobox默认情况下是不支持触屏上下滑动的,但可以通过自定义控件来实现这个功能。
一种常见的方法是使用自定义的控件去替代原生的Winform combobox控件。这个自定义控件可以继承自ComboBox,并重写其OnDropDown方法,以便在下拉菜单展开时添加一个滚动条。
具体步骤如下:
1. 创建一个新的Winform控件,取名为CustomComboBox。
2. 继承自ComboBox,并重写OnDropDown方法,以便在下拉菜单展开时添加一个滚动条。
```
public class CustomComboBox : ComboBox
{
protected override void OnDropDown(EventArgs e)
{
base.OnDropDown(e);
var handle = SendMessage(this.Handle, CB_GETCOMBOBOXINFO, IntPtr.Zero, IntPtr.Zero);
var info = (COMBOBOXINFO)Marshal.PtrToStructure(handle, typeof(COMBOBOXINFO));
var listBoxHandle = info.hwndList;
SetWindowLong(listBoxHandle, GWL_STYLE, GetWindowLong(listBoxHandle, GWL_STYLE) | LBS_NOTIFY | WS_VSCROLL);
}
private const int CB_GETCOMBOBOXINFO = 0x164;
private const int GWL_STYLE = -16;
private const int LBS_NOTIFY = 0x00000001;
private const int WS_VSCROLL = 0x00200000;
[DllImport("user32.dll", SetLastError = true)]
private static extern IntPtr SendMessage(IntPtr hWnd, int Msg, IntPtr wParam, IntPtr lParam);
[DllImport("user32.dll", SetLastError = true)]
private static extern int SetWindowLong(IntPtr hWnd, int nIndex, int dwNewLong);
[StructLayout(LayoutKind.Sequential)]
private struct COMBOBOXINFO
{
public int cbSize;
public RECT rcItem;
public RECT rcButton;
public int stateButton;
public IntPtr hwndCombo;
public IntPtr hwndEdit;
public IntPtr hwndList;
}
[StructLayout(LayoutKind.Sequential)]
private struct RECT
{
public int left, top, right, bottom;
}
}
```
3. 在Form中使用CustomComboBox,然后在TouchDown事件中记录当前触屏的坐标。
```
public partial class Form1 : Form
{
private Point _startPoint;
public Form1()
{
InitializeComponent();
}
private void customComboBox1_TouchDown(object sender, TouchEventArgs e)
{
_startPoint = e.Location;
}
}
```
4. 在TouchMove事件中计算当前触屏滑动的距离,然后根据距离调整下拉菜单的位置。
```
private void customComboBox1_TouchMove(object sender, TouchEventArgs e)
{
var distance = e.Location.Y - _startPoint.Y;
var dropDownHeight = customComboBox1.DropDownHeight;
if (distance > 0)
{
customComboBox1.DropDownHeight = Math.Min(dropDownHeight + distance, customComboBox1.Items.Count * customComboBox1.ItemHeight);
}
else
{
customComboBox1.DropDownHeight = Math.Max(dropDownHeight + distance, customComboBox1.ItemHeight);
}
}
```
5. 最后,在Form的Load事件中为CustomComboBox的TouchDown和TouchMove事件添加处理程序。
```
private void Form1_Load(object sender, EventArgs e)
{
customComboBox1.TouchDown += customComboBox1_TouchDown;
customComboBox1.TouchMove += customComboBox1_TouchMove;
}
```
这样,就可以在Winform中实现触屏上下滑动combobox的下拉部分了。
阅读全文
相关推荐



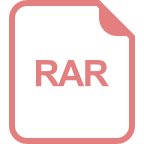












