os.walk
时间: 2023-06-29 13:20:30 浏览: 133
os.walk是Python中用于遍历文件夹的函数。它可以帮助我们递归遍历一个文件夹中的所有子文件夹和文件,并返回每一个文件夹路径、子文件夹列表和该文件夹下的所有文件列表。os.walk的使用方法如下:
``` python
import os
# 遍历文件夹,获取所有文件夹和文件的路径和名称
for root, dirs, files in os.walk("folder_path"):
# 输出当前正在遍历的文件夹路径
print("当前文件夹:", root)
# 输出当前文件夹中的子文件夹列表
print("子文件夹列表:", dirs)
# 输出当前文件夹中的所有文件名称
print("文件列表:", files)
```
在上面的代码中,"folder_path"是需要遍历的文件夹路径。os.walk会首先遍历该文件夹,然后递归遍历该文件夹中的所有子文件夹和文件,返回每一个文件夹路径、子文件夹列表和该文件夹下的所有文件列表。我们可以利用这些返回值来进一步处理文件夹和文件。
相关问题
79. 功能与下面程序段相同的是( ) import os path=os.getcwd() s=os.listdir(path) print(s) A. import os path=os.getcwd() s=os.walk(path) print(s) B. import os path=os.getcwd() s=os.walk(path) for dirpath,dirnames,filenames in s: print(filenames) C. import os path=os.getcwd() s=os.walk(path) for dirpath,dirnames,filenames in s: print(dirpath) D. import os path=os.getcwd() s=os.walk(path) for dirpath,dirnames,filenames in s: print(dirnames)
答案是 A。
程序段中,os.getcwd() 返回当前工作目录的路径,os.listdir(path) 返回指定目录下所有文件和子目录的名称列表,因此程序段的作用是列出当前工作目录下所有文件和子目录的名称列表。
选项 A 中的程序段与原程序段相同,os.walk(path) 也是递归地遍历指定目录及其子目录,并返回每个子目录中的文件和子目录的信息,但返回的信息形式不同,os.walk(path) 返回的是一个迭代器,可以通过遍历迭代器的方式获取每个子目录中的文件和子目录的详细信息。选项 B、C、D 中的程序段都是通过遍历 os.walk(path) 返回的迭代器来获取子目录中的文件和子目录的信息,只是打印的信息不同,因此这三个选项的功能与原程序段相同。
os.walk os.listdir
`os.walk` and `os.listdir` are both Python functions used to traverse directories and retrieve information about the files and subdirectories within them.
`os.walk` is a generator function that returns a tuple of three values for each directory it traverses: the path of the directory, a list of the subdirectories within that directory, and a list of the files within that directory. This function is often used to recursively traverse a directory tree and perform operations on all the files and directories within it.
Here's an example of using `os.walk` to print out the names of all the files in a directory tree:
```
import os
root_dir = '/path/to/root/dir'
for dirpath, dirnames, filenames in os.walk(root_dir):
for filename in filenames:
print(os.path.join(dirpath, filename))
```
`os.listdir`, on the other hand, is a simpler function that returns a list of all the files and directories within a given directory. It does not recursively traverse subdirectories, but only returns the names of the files and directories within the specified directory.
Here's an example of using `os.listdir` to print out the names of all the files in a directory:
```
import os
dir_path = '/path/to/dir'
for filename in os.listdir(dir_path):
if os.path.isfile(os.path.join(dir_path, filename)):
print(filename)
```
阅读全文
相关推荐
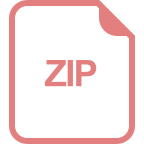
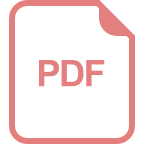
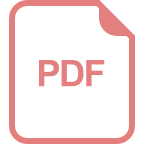
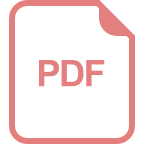











