c++文本文件新建及改写代码
时间: 2024-10-28 19:08:15 浏览: 18
在C++中,你可以使用`fstream`库来进行文件操作,包括创建、打开、读取和写入文本文件。以下是新建和改写文本文件的基本步骤:
```cpp
#include <fstream>
#include <string>
// 新建并清空文件
void createAndClearFile(const std::string& filename) {
std::ofstream file(filename);
if (file.is_open()) {
file.clear(); // 清除错误标志位
file.seekp(0); // 移动到文件开始
file.trunc(); // 设置文件长度为0
file.close();
std::cout << "文件 " << filename << " 创建成功并清空了内容." << std::endl;
} else {
std::cerr << "无法打开文件 " << filename << "." << std::endl;
}
}
// 追加内容到文件(如果存在则覆盖原有内容)
void rewriteFile(const std::string& filename, const std::string& content) {
std::ofstream file(filename, std::ios_base::trunc | std::ios_base::out); // 'trunc' 参数用于重置文件
if (file.is_open()) {
file << content;
file.close();
std::cout << "文件 " << filename << " 内容已更新." << std::endl;
} else {
std::cerr << "无法打开文件 " << filename << " 进行改写." << std::endl;
}
}
int main() {
createAndClearFile("test.txt");
rewriteFile("test.txt", "这是新的内容.");
return 0;
}
```
在这个例子中,`createAndClearFile`函数会创建一个新的文件,并将其长度设为0,相当于清空文件;而`rewriteFile`函数会打开指定的文件,如果文件存在,则先清空再写入新内容。
阅读全文
相关推荐






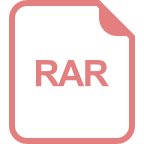
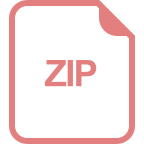
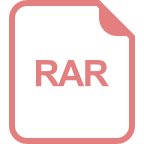
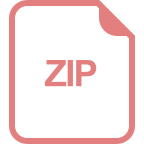


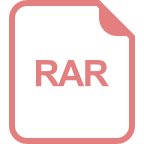

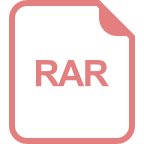


