如何用C++编写一个函数,将数组中的所有奇数和偶数分别进行排序?
时间: 2024-12-12 10:17:19 浏览: 12
在C++中,你可以创建两个独立的数组来分别存储奇数和偶数,然后分别对它们进行排序。以下是一个简单的示例,假设我们有一个整数数组:
```cpp
#include <algorithm>
#include <vector>
// Function to separate even and odd numbers into separate vectors
std::vector<int> separateAndSort(std::vector<int>& nums) {
std::vector<int> evens;
std::vector<int> odds;
// Iterate through the array and separate even and odd numbers
for (int num : nums) {
if (num % 2 == 0)
evens.push_back(num);
else
odds.push_back(num);
}
// Sort the two vectors using standard algorithms
std::sort(evens.begin(), evens.end());
std::sort(odds.begin(), odds.end());
// Return the sorted arrays concatenated
return {evens, odds};
}
int main() {
std::vector<int> input = {9, 7, 4, 2, 1, 6, 8, 3, 5};
auto result = separateAndSort(input);
// Print the sorted even and odd numbers
for (int num : result[0])
std::cout << "Even: " << num << " ";
std::cout << "\n";
for (int num : result[1])
std::cout << "Odd: " << num << " ";
return 0;
}
```
这个程序首先分离出奇数和偶数,然后使用`std::sort`函数对它们进行升序排列。注意这仅适用于原始数组允许复制的情况。如果你想原地操作,可以考虑使用`std::nth_element`等算法。
阅读全文
相关推荐
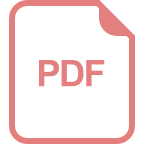
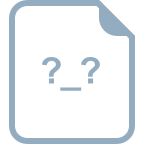
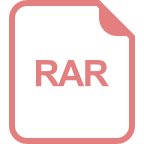















