JavaWeb连接SQLServer数据库并完成一个登录界面及其功能设计。
时间: 2024-02-23 22:00:05 浏览: 124
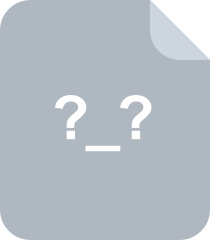
Java连接SQL数据库实现登陆

好的,我来回答你的问题。
首先,需要在JavaWeb项目中导入SQLServer的JDBC驱动,可以从官网下载对应版本的驱动包,将其拷贝到项目的lib目录下,并在项目的构建路径中添加。
接下来,需要在SQLServer中创建一个数据库,并在该数据库中创建一个用户表,用于存储用户的账号和密码信息。
在JavaWeb项目中,可以使用JSP页面作为登录界面,通过表单提交用户输入的账号和密码信息,将其传递到后台Servlet进行处理。在Servlet中,可以使用JDBC连接SQLServer数据库,根据用户输入的账号和密码查询用户表,验证用户身份是否正确。如果验证通过,则可以将用户信息保存到Session中,并跳转到主页面;否则,返回登录界面并提示用户输入的账号或密码错误。
以下是一个示例代码,仅供参考:
登录页面:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>登录页面</title>
</head>
<body>
<form action="login" method="post">
<label>账号:</label><input type="text" name="username"><br>
<label>密码:</label><input type="password" name="password"><br>
<input type="submit" value="登录">
</form>
</body>
</html>
```
登录Servlet:
```java
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
// 连接SQLServer数据库
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
conn = DriverManager.getConnection("jdbc:sqlserver://localhost:1433;DatabaseName=mydb", "sa", "123456");
// 查询用户表,验证用户身份
String sql = "SELECT * FROM user WHERE username=? AND password=?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, password);
rs = stmt.executeQuery();
if (rs.next()) {
// 用户身份验证通过,将用户信息保存到Session中
HttpSession session = request.getSession();
session.setAttribute("username", username);
// 跳转到主页面
response.sendRedirect("main.jsp");
} else {
// 用户身份验证失败,返回登录页面并提示错误信息
request.setAttribute("msg", "账号或密码错误");
request.getRequestDispatcher("login.jsp").forward(request, response);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
主页面:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>主页面</title>
</head>
<body>
<h1>欢迎,<%= session.getAttribute("username") %>!</h1>
<a href="logout">退出登录</a>
</body>
</html>
```
退出登录Servlet:
```java
@WebServlet("/logout")
public class LogoutServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 清空Session中保存的用户信息
HttpSession session = request.getSession();
session.invalidate();
// 跳转到登录页面
response.sendRedirect("login.jsp");
}
}
```
以上代码仅供参考,具体实现还需要根据自己的需求进行调整和完善。
阅读全文
相关推荐
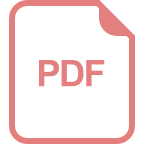

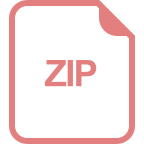
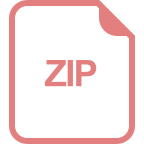
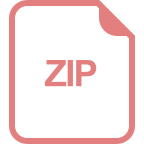
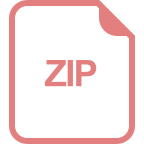
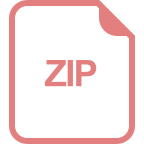
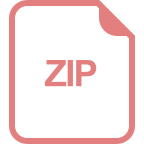
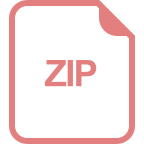
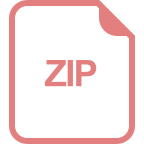
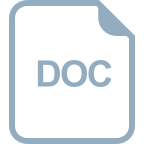
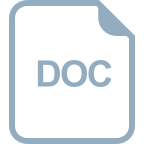
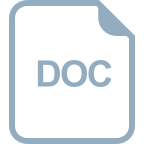
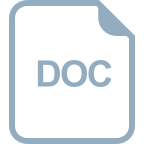
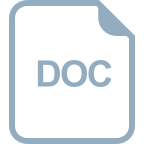
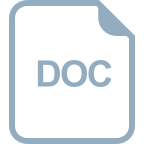