AttributeError: 'DataFrame' object has no attribute 'ftypes'. Did you mean: 'dtypes'?
时间: 2024-01-17 17:19:32 浏览: 271
这个错误是因为在代码中使用了`ftypes`属性,但是`DataFrame`对象没有`ftypes`属性。正确的属性应该是`dtypes`。你可以将代码中的`ftypes`改为`dtypes`来解决这个错误。
以下是修改后的代码示例:
```python
columns = df.columns.tolist()
field = [] # 用来接收字段名称的列表
table = [] # 用来接收字段名称和字段类型的列表
types = df.dtypes
print(types)
for col in columns:
if 'int' in str(df[col].dtype):
char = col + ' INT'
elif 'float' in str(df[col].dtype):
char = col + ' FLOAT'
elif 'object' in str(df[col].dtype):
char = col + ' VARCHAR(255)'
elif 'datetime' in str(df[col].dtype):
char = col + ' DATETIME'
else:
char = col + ' VARCHAR(255)'
table.append(char)
field.append(col)
```
相关问题
AttributeError: DataFrame object has no attribute append . Did you mean: _append ?
This error occurs when you try to call the `append` method on a Pandas DataFrame object, but the object does not have an `append` attribute.
One possible reason for this error is that you are trying to append a DataFrame to another DataFrame using the `append` method, but you are not using it correctly. In Pandas, the `append` method does not modify the original DataFrame, but instead it returns a new DataFrame that contains the rows from both DataFrames. Therefore, you need to assign the result of the `append` method to a new variable or to the original DataFrame, like this:
```
df1 = pd.DataFrame({'A': [1, 2], 'B': [3, 4]})
df2 = pd.DataFrame({'A': [5, 6], 'B': [7, 8]})
df3 = df1.append(df2)
```
In this example, `df3` will contain the rows from both `df1` and `df2`.
If you are still getting the AttributeError, it's possible that the DataFrame object you are trying to append to does not have an `append` attribute. In this case, you can try using the `_append` attribute instead, which is a private method that is used internally by Pandas. However, be aware that using private methods may not be supported in future versions of Pandas and may cause unexpected behavior.
```
df1._append(df2)
```
AttributeError: 'DataFrame' object has no attribute 'dtype'. Did you mean: 'dtypes'?
Yes, the error message "AttributeError: 'DataFrame' object has no attribute 'dtype'" suggests that you are trying to access the "dtype" attribute of a DataFrame object in Python, but it does not exist.
The correct attribute to access the data types of columns in a pandas DataFrame is "dtypes" (with an "s" at the end). So, you can try using `df.dtypes` instead of `df.dtype`.
For example:
```python
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': ['a', 'b', 'c']})
# Access the data types of columns
print(df.dtypes)
```
This will print the data types of each column in the DataFrame.
阅读全文
相关推荐
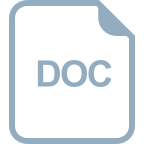
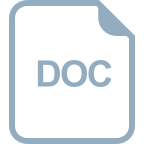
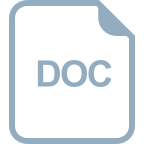













