C语言中如何能去除多余的空格
时间: 2024-10-18 19:10:32 浏览: 49
在C语言中,要去除字符串中的多余空格,你可以采用几种方法:
1. **使用`strtok()`函数**:这是一个线性扫描的方法,将字符串分解成单词。首先,你可以遍历整个字符串,找到连续的空格,并替换为空字符(`'\0'`)。例如:
```c
char *delSpaces(char *str) {
while (isspace(*str)) str++; // 跳过开头的空格
for (char *word = str; *word; word++) { // 找到单词边界
if (*word == ' ') { // 如果遇到空格
while (*(word+1) && isspace(*(word+1))) word++; // 跳过多余的空格
*word++ = '\0'; // 替换为结束符
}
}
return str;
}
```
2. **使用`str_replace()`函数**(如果是在有字符串处理库的情况下):
```c
#include <string.h>
void remove_spaces(char *str) {
char temp[strlen(str) + 1];
strcpy(temp, str);
int j = 0;
for (int i = 0; temp[i]; i++) {
if (!isspace(temp[i])) {
temp[j++] = temp[i];
}
}
temp[j] = '\0'; // 结束字符串
str = temp;
}
```
3. **使用指针操作**:
```c
void trimSpaces(char* str) {
char* start = str;
while (isspace(*start)) start++;
char* end = str + strlen(str) - 1;
while (end > start && isspace(*end)) end--;
*end + 1 = '\0';
}
```
以上方法都可以帮助你从字符串中删除多余的空格,然后通过返回新位置或直接改变原始字符串(如果你允许这样做)来获取处理后的结果。
阅读全文
相关推荐
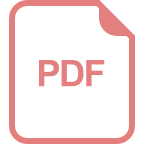
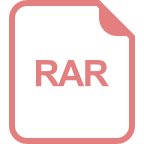
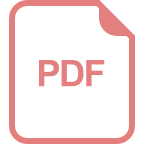















