vue3 获取数组最大值
时间: 2024-05-02 18:15:13 浏览: 11
在Vue3中,获取数组的最大值可以通过以下几种方式实现:
1. 使用Math.max()函数:可以通过将数组展开为参数的方式,使用Math.max()函数获取数组的最大值。例如:
```javascript
const arr = [1, 2, 3, 4, 5];
const max = Math.max(...arr);
console.log(max); // 输出:5
```
2. 使用reduce()方法:可以使用reduce()方法来迭代数组,并在每次迭代时比较当前值与累加器的值,从而得到最大值。例如:
```javascript
arr = [1, 2, 3, 4, 5];
const max = arr.reduce((acc, cur) => {
return Math.max(acc, cur);
});
console.log(max); // 输出:5
`
3. 使用扩展运算符和Math.max()函数结合:可以使用扩展运算符将数组展开为参数,并结合Math.max()函数获取最大值。例如:
```javascript
const arr = [1, 2, 3, 4, 5];
const max = Math.max(...arr);
console.log(max); // 输出:5
```
相关问题
vue从数组中获取最大的值
你可以使用 JavaScript 的 `Math.max()` 方法结合 `spread` 运算符来获取数组中的最大值。具体实现如下:
```javascript
const arr = [1, 3, 5, 2, 4];
const max = Math.max(...arr);
console.log(max); // 输出 5
```
如果你需要在 Vue 模板中获取数组中的最大值,你可以在计算属性中实现这个功能,例如:
```html
<template>
<div>
<p>数组: {{ arr }}</p>
<p>最大值: {{ max }}</p>
</div>
</template>
<script>
export default {
data() {
return {
arr: [1, 3, 5, 2, 4]
};
},
computed: {
max() {
return Math.max(...this.arr);
}
}
};
</script>
```
这样,当 `arr` 数组中的值发生变化时,计算属性 `max` 也会自动更新。
vue 随机获取数组中的数据
可以使用 Math.random() 方法生成一个随机数,然后将数组的长度作为最大值,用 Math.floor() 方法将随机数向下取整,得到一个随机的数组下标,最后返回该下标对应的数组元素即可。具体代码实现可以参考以下示例:
let arr = [1, 2, 3, 4, 5];
let randomIndex = Math.floor(Math.random() * arr.length);
let randomItem = arr[randomIndex];
console.log(randomItem); // 随机输出数组中的一个元素
相关推荐
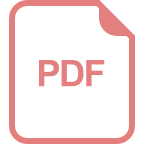












