这里面的每一道题我应该怎样写
时间: 2024-12-25 19:32:44 浏览: 4
根据提供的实验报告内容,你可以按照以下步骤来完成每个部分的任务:
### 1. 创建带时期索引的对象
#### (1) 创建 Period 对象
- **任务**:创建 `Period` 对象,表示特定的时间段。
- **具体步骤**:
- 使用 `pd.Period()` 函数创建两个 `Period` 对象:
- 第一个对象表示从 `2024-01-01` 到 `2024-12-31` 的年份。
- 第二个对象表示从 `2024-11-01` 到 `2024-11-30` 的月份。
```python
import pandas as pd
period_year = pd.Period('2024', 'A')
period_month = pd.Period('2024-11', 'M')
```
#### (2) 时期计算
- **任务**:对 `Period` 对象进行加减操作。
- **具体步骤**:
- 将上述创建的 `Period` 对象分别加上和减去一个整数 2。
- 创建一个新的 `Period` 对象,使其频率与 `period_month` 相同,并进行数据运算。
```python
period_year_plus_2 = period_year + 2
period_year_minus_2 = period_year - 2
period_month_plus_2 = period_month + 2
period_month_minus_2 = period_month - 2
new_period = pd.Period('2024-11', 'M')
result = period_month + new_period
```
#### (3) 创建 PeriodIndex 类的对象
- **任务**:创建带有固定频率的 `PeriodIndex` 对象。
- **具体步骤**:
- 使用 `pd.period_range()` 方法创建多个固定频率的 `Period` 对象。
- 直接调用 `pd.PeriodIndex()` 方法,传入一组日期字符串和频率。
```python
period_index_range = pd.period_range(start='2024-01-01', end='2024-12-31', freq='M')
date_strings = ['2024-01-01', '2024-02-01', '2024-03-01']
period_index_direct = pd.PeriodIndex(date_strings, freq='M')
```
#### (4) 创建 Series 类的对象
- 创建一个数据集。
- 使用 `pd.Series()` 方法创建 `Series` 对象,其中 `data` 是生成的数据,`index` 是 `PeriodIndex`。
```python
data = [i for i in range(len(period_index_range))]
series = pd.Series(data, index=period_index_range)
```
### 2. 重采样
#### (1) 创建 DatetimeIndex 对象
- **任务**:创建一个包含 100 个时间戳的 `DatetimeIndex` 对象。
- **具体步骤**:
- 使用 `pd.date_range()` 方法创建时间戳。
```python
datetime_index = pd.date_range(start='2024-11-29', periods=100, freq='H')
```
#### (2) 创建数据集
- **任务**:生成一个随机数据集。
- **具体步骤**:
- 使用 `np.random.rand()` 方法生成随机数据。
```python
import numpy as np
random_data = np.random.rand(100)
```
#### (3) 创建 Series 对象
- **任务**:创建一个 `Series` 对象,其索引为 `DatetimeIndex`。
- **具体步骤**:
- 使用 `pd.Series()` 方法创建 `Series` 对象。
```python
series = pd.Series(random_data, index=datetime_index)
```
#### (4) 重新采样
- **任务**:按周重采样。
- **具体步骤**:
- 使用 `resample()` 方法按周重采样。
```python
weekly_sampled = series.resample('W').mean()
```
#### (5) 降采样
- **任务**:通过 `resample()` 和 `ohlc()` 方法实现降采样。
- **具体步骤**:
- 使用 `resample()` 方法按 7 天降采样,并使用 `ohlc()` 方法获取高开低收数据。
- 通过分组实现降采样,取平均值。
```python
ohlc_downsampled = series.resample('7D').ohlc()
grouped = series.groupby(pd.Grouper(freq='7D'))
mean_downsampled = grouped.mean()
```
#### (6) 升采样
- **任务**:将降采样后的数据升采样。
- **具体步骤**:
- 使用 `resample()` 方法按天升采样,并使用 `asfreq()` 方法填充缺失值。
```python
upsampled = ohlc_downsampled.resample('D').asfreq().ffill(limit=2)
```
### 3. 滑动窗口
#### (1) 创建数据集
- **任务**:创建一个数据集,取值范围为 `[20, 90]` 之间的整数,长度为 100。
- **具体步骤**:
- 使用 `np.random.randint()` 方法生成数据。
```python
data = np.random.randint(20, 91, size=100)
```
#### (2) 创建 DatetimeIndex 对象
- **任务**:创建一个包含 100 个时间戳的 `DatetimeIndex` 对象。
- **具体步骤**:
- 使用 `pd.date_range()` 方法创建时间戳。
```python
datetime_index = pd.date_range(start='2024-11-29', periods=100, freq='H')
```
#### (3) 创建 Series 对象
- **任务**:创建一个 `Series` 对象。
- **具体步骤**:
- 使用 `pd.Series()` 方法创建 `Series` 对象。
```python
series = pd.Series(data, index=datetime_index)
```
#### (4) 创建滑动窗口
- **任务**:创建一个滑动窗口,长度为 5。
- **具体步骤**:
- 使用 `rolling()` 方法创建滑动窗口。
```python
window = series.rolling(window=5)
```
#### (5) 计算平均值
- **任务**:在时间窗口中计算平均值。
- **具体步骤**:
- 使用 `mean()` 方法计算平均值。
```python
rolling_mean = window.mean()
```
#### (6) 绘制折线图
- **任务**:绘制原始数据与滑动窗口数据的折线图。
- **具体步骤**:
- 使用 `plot()` 方法绘制折线图。
```python
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
series.plot(ax=ax, label='Original Data')
rolling_mean.plot(ax=ax, label='Rolling Mean')
ax.legend()
plt.show()
```
希望这些步骤能帮助你顺利完成实验报告中的各个任务!如果有任何疑问或需要进一步的帮助,请随时提问。
阅读全文
相关推荐













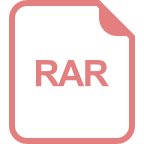
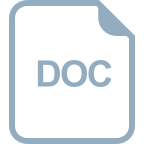


