springboot流式视频播放
时间: 2023-10-17 21:34:03 浏览: 62
在Spring Boot中实现流式视频播放可以通过以下步骤完成:
1. 首先,确保你的Spring Boot项目中已经引入了Web和Thymeleaf依赖。可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
```
2. 创建一个控制器类,用于处理视频请求和流式传输。在该类上使用`@RestController`注解:
```java
@RestController
public class VideoController {
@RequestMapping(value = "/video", method = RequestMethod.GET, produces = "video/mp4")
public ResponseEntity<InputStreamResource> streamVideo() throws IOException {
// 通过IO流读取视频文件
InputStream videoFileStream = new FileInputStream("path/to/video.mp4");
// 创建流式传输资源对象
InputStreamResource videoStreamResource = new InputStreamResource(videoFileStream);
// 返回响应实体,包含视频流和Content-Length头
return ResponseEntity
.ok()
.contentLength(videoFileStream.available())
.contentType(MediaType.parseMediaType("video/mp4"))
.body(videoStreamResource);
}
}
```
3. 在上述代码中,需要将`"path/to/video.mp4"`替换为实际视频文件的路径。注意,视频文件必须存在于指定路径。
4. 在Thymeleaf模板中创建一个视频播放页面。创建一个名为`play.html`的文件,并在其中添加以下代码:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Video Player</title>
</head>
<body>
<video width="640" height="480" controls>
<source th:src="@{/video}" type="video/mp4">
</video>
</body>
</html>
```
5. 启动Spring Boot应用程序,访问`http://localhost:8080/play`即可在浏览器中播放视频。
注意:请将视频文件的实际路径替换为你的视频文件路径,并确保视频文件存在。另外,确保视频文件格式与上述示例代码中指定的Content-Type一致(这里是"video/mp4")。
这样,你就可以在Spring Boot中实现流式视频播放了。
相关推荐
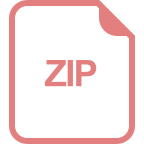














