python购物车结算
时间: 2024-06-18 14:01:12 浏览: 179
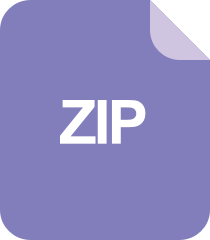
【python面向对象】购物车系统,文件有两个版本,进行结算与支付功能,帮助初学者更好的掌握从面向过程向面向对象过渡。
在Python中实现一个简单的购物车结算功能,通常涉及到以下几个步骤:
1. **创建商品类**:定义一个商品类(例如`Product`),包含属性如名称、价格、数量等。
```python
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
self.quantity = 0
def set_quantity(self, quantity):
self.quantity = quantity
def get_total_cost(self):
return self.price * self.quantity
```
2. **购物车类**:创建一个购物车类(例如` ShoppingCart`),用于存储商品和处理结算。
```python
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, product, quantity=1):
item = product
item.set_quantity(quantity)
self.items.append(item)
def remove_item(self, product_name, quantity=None):
for item in self.items:
if item.name == product_name:
if quantity is not None:
item.set_quantity(item.quantity - quantity)
else:
self.items.remove(item)
break
def total_cost(self):
return sum(item.get_total_cost() for item in self.items)
```
3. **使用示例**:
```python
# 创建商品实例
apple = Product("Apple", 1.5)
banana = Product("Banana", 0.5)
# 添加商品到购物车
cart = ShoppingCart()
cart.add_item(apple, 3)
cart.add_item(banana, 2)
# 结算
print(f"Total cost: {cart.total_cost():.2f}")
# 删除商品
cart.remove_item("Apple", 1)
print(f"New total cost after removing an apple: {cart.total_cost():.2f}")
```
这个例子中,购物车可以添加商品,查询总成本,以及删除特定商品。你可以根据需求扩展购物车的功能,比如计算优惠后的总价、查看购物车内容等。
阅读全文
相关推荐
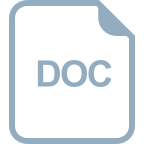
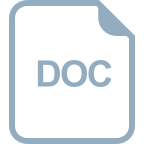
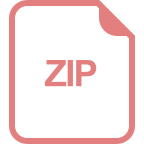
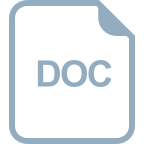




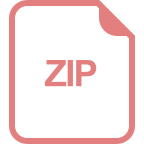
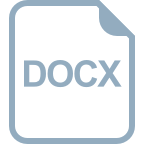
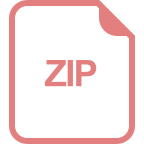
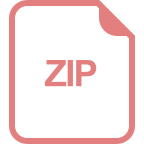
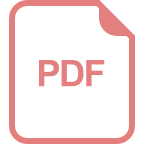




