vue3 的 props
时间: 2023-11-01 11:08:29 浏览: 40
Vue 3中的props与Vue 2中的有一些区别。首先,Vue 3中的props默认是可选的,除非你将其声明为`required: true`。对于非布尔类型的未传递的可选props,默认值将会是`undefined`。而对于布尔类型的未传递props,默认值将会被转换为`false`。你可以通过设置`default`来改变这个行为,比如将其设置为`default: undefined`,这样就与非布尔类型的props的行为保持一致了。
在Vue 3中,当props的值被解析为`undefined`时,不论是未被传递还是显式指定为`undefined`,它都会被改为默认值。如果props的验证失败,Vue会在开发模式下抛出一个控制台警告。
总结来说,Vue 3中的props具有更灵活的默认值设置和校验功能,使得传递和使用props更加方便和可靠。
相关问题
Vue3 props
在Vue3中,可以使用`props`属性来接收父组件传递的数据。你可以通过在子组件的`props`选项中指定属性的名称来声明需要接收的属性。例如,如果父组件传递了一个名为`title`的属性,你可以通过在子组件的`props`选项中指定`title`来接收该属性。例如:
```javascript
props: ['title']
```
在子组件的模板中,你可以直接使用`title`来访问父组件传递过来的数据。例如:
```html
<template>
<h2>{{ title }}</h2>
</template>
```
如果你需要在`setup`函数中使用props,你可以将其作为第一个参数传递。例如:
```javascript
setup(props) {
console.log(props.title)
}
```
通过这种方式,你可以在子组件中接收和使用父组件传递的props数据。<em>1</em><em>2</em><em>3</em>
#### 引用[.reference_title]
- *1* [Vue 3.0学习笔记之 Props](https://blog.csdn.net/wm201316/article/details/128877166)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}} ] [.reference_item]
- *2* *3* [vue3父子组件传值,props使用细节](https://blog.csdn.net/weixin_45304198/article/details/120257562)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}} ] [.reference_item]
[ .reference_list ]
vue3 props
Vue3中props和emits的使用方法:
props:
1. 在组件中声明props,例如:props: { title: String }
2. 在使用组件时,通过设置组件的属性来传递数据,例如:<my-component title="Hello World"></my-component>
emits:
1. 在组件内部,使用$emit触发事件,例如:this.$emit('myEvent', data)
2. 在使用组件时,通过绑定事件来监听该事件,例如:<my-component @myEvent="handleMyEvent"></my-component>
相关推荐
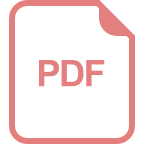













