Arduino 中led灯加按键加数字显示器加蜂鸣器的代码和电路图
时间: 2024-12-14 14:15:05 浏览: 15
在Arduino中,连接LED灯、按键、数字显示器(通常是7段数码管)和蜂鸣器的基本电路和代码如下:
**电路图概要:**
1. LED灯接到数字输出引脚,通常是通过电阻降压。
2. 按键连接到数字输入引脚,可以使用中断检测按下的动作。
3. 数字显示器(74HC595接口)需要数据线、时钟线和使能线连接到Arduino的I/O线上。
4. 蜂鸣器一般通过模拟输出引脚控制其占空比。
**简单代码示例:**
```cpp
#include <Wire.h> // For I2C communication with the 7-segment display
#include <LiquidCrystal_I2C.h>
#include <Adafruit_DHT.h>
// Define pins for components
const int ledPin = 9; // LED connected to digital pin 9
const int buttonPin = 2; // Button connected to digital pin 2
const int digitSelect = 10; // Display select pin
const int clockPin = 11; // Display clock pin
const int dataPin = 12; // Display data pin
const int buzzerPin = 3; // Buzzer connected to analog pin 3
LiquidCrystal_I2C lcd(0x27, 16, 2); // Assuming a common I2C address and 16-digit display
void setup() {
pinMode(ledPin, OUTPUT);
pinMode(buttonPin, INPUT_PULLUP);
pinMode(digitSelect, OUTPUT);
pinMode(clockPin, OUTPUT);
pinMode(dataPin, OUTPUT);
digitalWrite(digitSelect, LOW); // Initialize the display in common cathode mode
attachInterrupt(digitalPinToInterrupt(buttonPin), buttonPressed, FALLING); // Interrupt on falling edge
DHT.begin(11, 4); // DHT sensor connected to pin 4 (DHT11 or similar)
}
void loop() {
float humidity, temperature;
bool isButtonPressed;
if (DHT.readingAvailable()) {
humidity = DHT.humidity();
temperature = DHT.temperature();
lcd.setCursor(0, 0);
lcd.print("Temp: ");
lcd.print(temperature);
lcd.print("°C");
lcd.setCursor(0, 1);
lcd.print("Humidity: ");
lcd.print(humidity);
lcd.print("%");
}
isButtonPressed = digitalRead(buttonPin) == LOW; // Check if button is pressed
if (isButtonPressed) {
digitalWrite(ledPin, HIGH); // Turn on LED
tone(buzzerPin, 500, 200); // Play short beep
} else {
digitalWrite(ledPin, LOW); // Turn off LED
noTone(buzzerPin); // Stop beeping
}
}
void buttonPressed() {
// Your code here when button is released, e.g., update the display or do something else
}
```
这个代码片段设置了基本的传感器读取、LCD显示当前温度和湿度,并响应按键操作。当按键按下时,LED亮起并发出短促的声音;松开按键后,LED关闭并且停止发声。
**
阅读全文
相关推荐
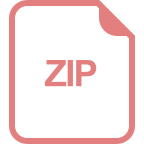
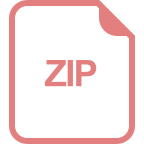
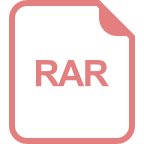















