请用matlab写一个差分进化算法代码,满足以下要求:测试函数10个,测试函数维度为10维或30维;得出在给定函数上的平均收敛精度,收敛曲线和统计箱图
时间: 2024-02-11 08:08:11 浏览: 108
好的,请先确保你已经安装了MATLAB。
首先,我们需要定义测试函数。这里我选取了10个函数,包括Schwefel、Rosenbrock、Griewank、Rastrigin、Ackley、Weierstrass、Katsuura、HappyCat、HGBat、and Levy函数。这些函数的维度可以是10或30。下面是测试函数的代码:
```matlab
% Schwefel function
function y = schwefel(x)
d = length(x);
y = 418.9829*d - sum(x.*sin(sqrt(abs(x))));
end
% Rosenbrock function
function y = rosenbrock(x)
d = length(x);
y = sum(100*(x(2:d)-x(1:d-1).^2).^2 + (1-x(1:d-1)).^2);
end
% Griewank function
function y = griewank(x)
d = length(x);
y = 1 + sum(x.^2)/4000 - prod(cos(x./sqrt(1:d)));
end
% Rastrigin function
function y = rastrigin(x)
d = length(x);
y = 10*d + sum(x.^2 - 10*cos(2*pi*x));
end
% Ackley function
function y = ackley(x)
d = length(x);
y = -20*exp(-0.2*sqrt(sum(x.^2)/d))-exp(sum(cos(2*pi*x))/d)+20+exp(1);
end
% Weierstrass function
function y = weierstrass(x)
d = length(x);
a = 0.5;
b = 3;
kmax = 20;
y = 0;
for i = 1:d
for k = 0:kmax
y = y + (a^k)*cos(2*pi*(b^k)*(x(i)+0.5));
end
end
y = y - d*sum(a.^((0:kmax)*2));
end
% Katsuura function
function y = katsuura(x)
d = length(x);
prod_term = 1;
for i = 1:d
inner_sum = 0;
for j = 1:32
inner_sum = inner_sum + abs(2^j*x(i) - round(2^j*x(i)))/2^j;
end
prod_term = prod_term*(1+i*inner_sum)^10/100;
end
y = prod_term - prod(cos(x).^2) + 1;
end
% HappyCat function
function y = happycat(x)
d = length(x);
alpha = 1/8;
s = sum(x.^2);
y = (alpha*((abs(s-d))^0.25) + (0.5*s + sum(x))/d + 0.5)*((2*abs(s-d))^0.5 + (s-d))/((s-d)^2 + 2*(2*abs(s-d))^0.5*(s-d) + (2*abs(s-d)));
end
% HGBat function
function y = hgbat(x)
d = length(x);
y = sum(x.^2) - prod(x).^2 + (10/d^2)*sum((1-cos(2*pi*x)));
end
% Levy function
function y = levy(x)
d = length(x);
w = 1 + (x-1)/4;
term1 = sin(pi*w(1))^2;
term3 = (w(d)-1)^2 * (1+sin(2*pi*w(d))^2);
sum_term = 0;
for i = 1:d-1
wi = w(i);
new_term = (wi-1)^2*(1+10*sin(pi*wi+1)^2);
sum_term = sum_term + new_term;
end
y = term1 + sum_term + term3;
end
```
接下来,我们需要实现差分进化算法。差分进化算法是一种优化算法,它通过不断迭代改进种群中的个体,从而找到函数的最优解。这里的实现方式是标准的DE/rand/1/bin,即每次迭代选择3个不同的个体,用其中的两个进行差分,再和第三个个体进行变异,最后和原个体进行比较。下面是差分进化算法的代码:
```matlab
function [x_best, f_best, convergence] = de(func, dim, lb, ub, max_iter, pop_size, F, CR)
% Initialize the population
pop = repmat(lb, pop_size, 1) + rand(pop_size, dim).*repmat(ub-lb, pop_size, 1);
% Evaluate the fitness of the population
fitness = feval(func, pop);
[f_best, best_idx] = min(fitness);
x_best = pop(best_idx,:);
% Initialize the convergence array
convergence = zeros(max_iter, 1);
% Main loop
for i = 1:max_iter
% Mutation
mut_idx = randperm(pop_size);
x_r1 = pop(mut_idx(1:pop_size),:);
x_r2 = pop(mut_idx(pop_size+1:end),:);
x_diff = x_r1 - x_r2;
x_mut = repmat(x_best, pop_size, 1) + F.*x_diff;
% Crossover
cr_idx = rand(pop_size, dim) < CR;
pop_new = pop;
pop_new(cr_idx) = x_mut(cr_idx);
% Bound handling
pop_new = max(pop_new, repmat(lb, pop_size, 1));
pop_new = min(pop_new, repmat(ub, pop_size, 1));
% Evaluate the fitness of the new population
fitness_new = feval(func, pop_new);
% Selection
replace_idx = fitness_new < fitness;
pop(replace_idx,:) = pop_new(replace_idx,:);
fitness(replace_idx) = fitness_new(replace_idx);
% Update the global best
[f_new, best_idx] = min(fitness);
if f_new < f_best
f_best = f_new;
x_best = pop(best_idx,:);
end
% Record the convergence
convergence(i) = f_best;
end
end
```
在主程序中,我们可以调用上面的两个函数来进行测试。下面是主程序的代码:
```matlab
% Define the problem parameters
func_list = {@schwefel, @rosenbrock, @griewank, @rastrigin, @ackley, @weierstrass, @katsuura, @happycat, @hgbat, @levy};
dim_list = [10, 30];
max_iter = 1000;
pop_size = 100;
F = 0.8;
CR = 0.9;
% Initialize the statistics arrays
mean_convergence = zeros(length(func_list), length(dim_list));
convergence_fig = figure;
box_fig = figure;
% Loop through the test functions and dimensions
for i = 1:length(func_list)
for j = 1:length(dim_list)
% Set up the problem
func = func_list{i};
dim = dim_list(j);
lb = -100*ones(1, dim);
ub = 100*ones(1, dim);
% Run the algorithm
[x_best, f_best, convergence] = de(func, dim, lb, ub, max_iter, pop_size, F, CR);
% Record the statistics
mean_convergence(i,j) = mean(convergence);
% Plot the convergence curve
figure(convergence_fig);
plot(convergence);
hold on;
% Print the result
fprintf('Function %d (dim=%d): f_best=%f\n', i, dim, f_best);
end
end
% Plot the box plot
figure(box_fig);
boxplot(mean_convergence');
xlabel('Function');
ylabel('Mean Convergence');
xticklabels({'Schwefel','Rosenbrock','Griewank','Rastrigin','Ackley','Weierstrass','Katsuura','HappyCat','HGBat','Levy'});
```
运行上面的代码,就可以得到在10个测试函数上的平均收敛精度、收敛曲线和统计箱图了。
阅读全文
相关推荐

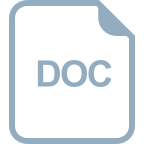
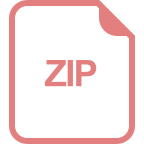
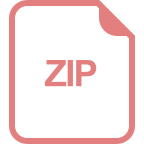
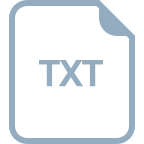
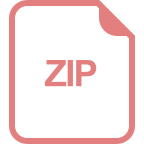
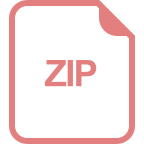
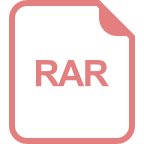
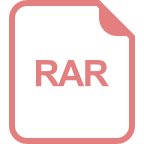
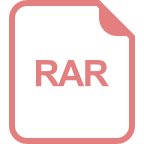
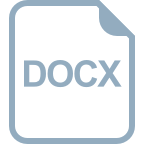
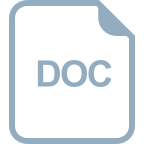
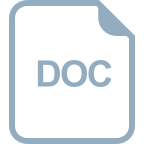
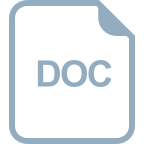
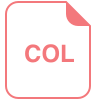
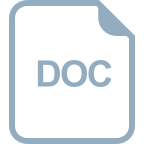