python pyqt5编写一个界面,能够入excel数据并通过新打开界面对数据进行增删改查
时间: 2024-02-13 14:04:09 浏览: 212
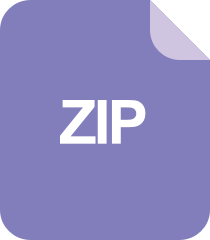
python+PyQt5 Excel小工具开发
好的,我可以为您提供一个基本的PyQt5界面示例,它可以打开Excel文件并将数据显示在表格视图中。您可以使用该界面来对数据进行增删改查。请按照以下步骤操作:
1. 安装PyQt5和pandas库
您可以使用以下命令在命令行中安装这些库:
```python
pip install pyqt5 pandas
```
2. 创建一个新的PyQt5应用程序
您可以使用以下代码创建一个新的PyQt5应用程序:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
app = QApplication(sys.argv)
window = QMainWindow()
window.show()
sys.exit(app.exec_())
```
3. 添加一个按钮和文件对话框
您可以使用以下代码向窗口添加一个按钮和一个文件对话框:
```python
from PyQt5.QtWidgets import QPushButton, QFileDialog
button = QPushButton('打开文件', window)
button.clicked.connect(lambda: open_file_dialog())
def open_file_dialog():
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_name, _ = QFileDialog.getOpenFileName(window, "打开文件", "", "Excel Files (*.xls *.xlsx)", options=options)
if file_name:
print(file_name)
```
4. 使用pandas读取Excel文件并将数据显示在表格视图中
您可以使用以下代码使用pandas读取Excel文件并将数据显示在表格视图中:
```python
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QColor
from PyQt5.QtWidgets import QTableView
import pandas as pd
table_view = QTableView(window)
table_view.setSortingEnabled(True)
def open_file_dialog():
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_name, _ = QFileDialog.getOpenFileName(window, "打开文件", "", "Excel Files (*.xls *.xlsx)", options=options)
if file_name:
df = pd.read_excel(file_name)
model = PandasModel(df)
table_view.setModel(model)
class PandasModel(QAbstractTableModel):
def __init__(self, data):
QAbstractTableModel.__init__(self)
self._data = data
def rowCount(self, parent=None):
return len(self._data.values)
def columnCount(self, parent=None):
return self._data.columns.size
def data(self, index, role=Qt.DisplayRole):
if index.isValid():
if role == Qt.DisplayRole or role == Qt.EditRole:
return str(self._data.values[index.row()][index.column()])
elif role == Qt.BackgroundRole:
return QColor(Qt.white)
return None
def headerData(self, section, orientation, role):
if role == Qt.DisplayRole and orientation == Qt.Horizontal:
return self._data.columns[section]
return QAbstractTableModel.headerData(self, section, orientation, role)
```
此示例创建一个新的表格模型类`PandasModel`,该类继承自`QAbstractTableModel`类。该模型类使用pandas库读取Excel文件并将数据存储在一个pandas数据帧中。然后,该模型类将数据帧中的数据显示在表格视图中。
现在,您可以使用该界面来打开Excel文件并将数据显示在表格视图中。您可以使用pandas库进行数据处理,并通过该界面将更改保存回Excel文件。
阅读全文
相关推荐
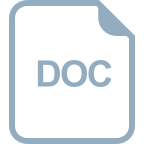
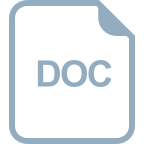

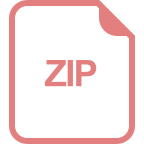
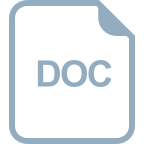
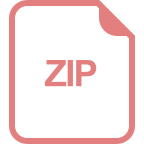
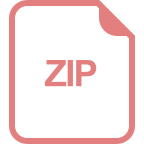
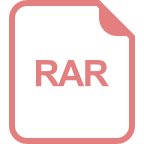
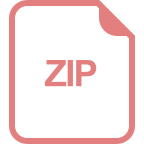
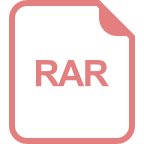
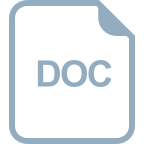
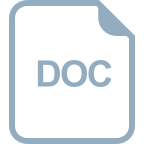
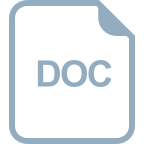
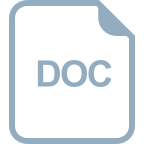
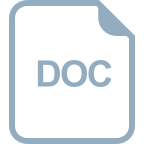


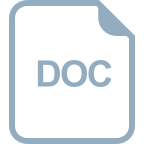