pygame绘制走势图
时间: 2023-09-02 08:11:38 浏览: 52
要使用 Pygame 绘制走势图,您需要考虑以下步骤:
1. 准备数据集:首先,您需要准备一组数据,这些数据将在走势图中绘制。您可以使用 NumPy 等 Python 库生成随机数据或从文件中读取数据。
2. 设计窗口:使用 Pygame 库创建一个窗口,该窗口将显示您的走势图。您可以根据需要设置窗口的大小和颜色。
3. 绘制坐标轴:在窗口中,您需要绘制一个坐标轴,该坐标轴将显示您的数据。您可以使用 Pygame 提供的绘制直线和文本功能来绘制坐标轴。
4. 绘制数据:使用绘制直线功能,您可以将数据绘制在坐标轴上。您可以使用不同的颜色和线条样式来表示不同的数据。
5. 显示图形:将所有元素绘制到窗口上后,您需要使用 Pygame 的显示函数来显示图形。
下面是一个简单的示例代码,可以帮助您开始绘制走势图:
```python
import pygame
import numpy as np
# 准备数据
data = np.random.randint(0, 100, size=(50))
# 设置窗口大小
width, height = 800, 600
# 初始化 Pygame
pygame.init()
# 创建窗口
screen = pygame.display.set_mode((width, height))
# 设置窗口标题
pygame.display.set_caption("Trend Chart")
# 设置颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
# 绘制坐标轴
def draw_axis():
pygame.draw.line(screen, BLACK, (50, height-50), (width-50, height-50), 2)
pygame.draw.line(screen, BLACK, (50, height-50), (50, 50), 2)
for i in range(10):
x = i * (width-100) / 9 + 50
pygame.draw.line(screen, GRAY, (x, height-50), (x, 50), 1)
text = str(i * 10)
font = pygame.font.Font(None, 20)
text = font.render(text, True, BLACK)
text_rect = text.get_rect(center=(x, height-30))
screen.blit(text, text_rect)
# 绘制数据
def draw_data():
for i in range(len(data)-1):
x1 = i * (width-100) / (len(data)-1) + 50
y1 = height - data[i] * (height-100) / 100 - 50
x2 = (i+1) * (width-100) / (len(data)-1) + 50
y2 = height - data[i+1] * (height-100) / 100 - 50
pygame.draw.line(screen, WHITE, (x1, y1), (x2, y2), 2)
# 主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 填充背景色
screen.fill(WHITE)
# 绘制坐标轴和数据
draw_axis()
draw_data()
# 显示图形
pygame.display.flip()
# 退出 Pygame
pygame.quit()
```
该示例代码使用 Pygame 库创建一个窗口,并绘制随机数据的走势图。您可以根据需要修改代码以适应您的数据集和样式。
相关推荐
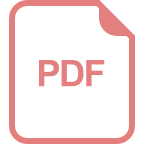
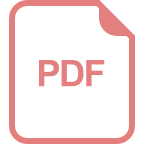
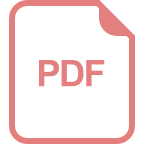














