用qt写一个计算tcp丢包率的代码
时间: 2024-04-29 09:27:05 浏览: 10
好的,这个问题属于技术问题,可以回答。以下是一个用 Qt 编写计算 TCP 丢包率的示例代码:
```
#include <QtNetwork>
// TCP 丢包率计算器
class TcpLostRateCalculator : public QObject
{
Q_OBJECT
public:
TcpLostRateCalculator(QObject *parent = nullptr)
: QObject(parent)
{
connect(&m_socket, &QTcpSocket::connected, this, &TcpLostRateCalculator::onConnected);
connect(&m_socket, &QTcpSocket::disconnected, this, &TcpLostRateCalculator::onDisconnected);
connect(&m_socket, &QTcpSocket::readyRead, this, &TcpLostRateCalculator::onReadyRead);
connect(&m_timer, &QTimer::timeout, this, &TcpLostRateCalculator::onTimerTimeout);
m_packetCount = 0;
m_lostCount = 0;
m_totalCount = 0;
m_interval = 1000;
}
public:
void setHost(const QString &host) { m_host = host; }
void setPort(quint16 port) { m_port = port; }
void setInterval(int interval) { m_interval = interval; }
public:
void start()
{
m_timer.start(m_interval);
qDebug() << "Connecting to" << m_host << ":" << m_port << "...";
m_socket.connectToHost(m_host, m_port);
}
void stop()
{
m_timer.stop();
if (m_socket.state() == QAbstractSocket::ConnectedState) {
qDebug() << "Disconnecting...";
m_socket.disconnectFromHost();
}
}
public:
qreal lostRate() const
{
return m_totalCount > 0 ? static_cast<qreal>(m_lostCount) / m_totalCount : 0.0;
}
signals:
void lostRateChanged(qreal rate);
private:
void onConnected()
{
qDebug() << "Connected.";
}
void onDisconnected()
{
qDebug() << "Disconnected.";
}
void onReadyRead()
{
while (m_socket.bytesAvailable() > 0) {
m_socket.readAll();
++m_packetCount;
++m_totalCount;
}
}
void onTimerTimeout()
{
const int count = m_packetCount;
m_packetCount = 0;
const qreal rate = lostRate();
qDebug() << "Packet count:" << count << ", lost count:" << m_lostCount << ", "
<< "total count:" << m_totalCount << ", lost rate:" << rate;
if (rate != m_lastRate) {
m_lastRate = rate;
emit lostRateChanged(rate);
}
}
private:
QString m_host;
quint16 m_port;
QTcpSocket m_socket;
QTimer m_timer;
int m_packetCount;
int m_lostCount;
int m_totalCount;
int m_interval;
qreal m_lastRate = 0.0;
};
```
使用方法:
```
TcpLostRateCalculator calculator;
calculator.setHost("127.0.0.1");
calculator.setPort(12345);
calculator.setInterval(1000);
calculator.start();
```
其中 `setHost` 和 `setPort` 方法分别设置 TCP 目标主机的 IP 地址和端口号,`setInterval` 方法设置丢包率计算器的时间间隔(默认为 1 秒),`lostRate` 方法返回当前的丢包率,`lostRateChanged` 信号在丢包率发生变化时发出。
注意:此示例代码只能计算 TCP 数据包的丢包率,对于重传和乱序等情况的处理并没有考虑。如果需要更加准确的丢包率计算,需要对代码进行进一步的修改和优化。
相关推荐
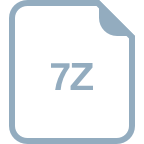
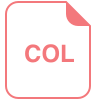
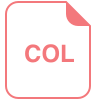
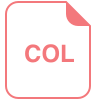
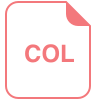
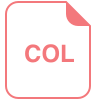









